利用qt基于ffmpeg写出一个视频播放界面,包括所有需要的文件
时间: 2024-02-11 18:05:34 浏览: 29
实现视频播放界面需要用到Qt和FFmpeg库。下面是一个简单的Qt视频播放器的示例代码,包括所有需要的文件。
首先,创建一个Qt项目,然后将以下文件添加到项目中:
1. main.cpp
```
#include "mainwindow.h"
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
```
2. mainwindow.h
```
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <QTimer>
#include "videoplayer.h"
namespace Ui {
class MainWindow;
}
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = 0);
~MainWindow();
private slots:
void on_actionOpen_triggered();
void updatePlayer();
private:
Ui::MainWindow *ui;
VideoPlayer *player;
QTimer *timer;
};
#endif // MAINWINDOW_H
```
3. mainwindow.cpp
```
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <QFileDialog>
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
player = new VideoPlayer(this);
ui->centralWidget->layout()->addWidget(player);
timer = new QTimer(this);
connect(timer, SIGNAL(timeout()), this, SLOT(updatePlayer()));
timer->start(40);
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::on_actionOpen_triggered()
{
QString fileName = QFileDialog::getOpenFileName(this, tr("Open Video"), "/", tr("Video Files (*.avi *.mp4 *.flv)"));
if (!fileName.isEmpty())
player->openFile(fileName);
}
void MainWindow::updatePlayer()
{
player->update();
}
```
4. videoplayer.h
```
#ifndef VIDEOPLAYER_H
#define VIDEOPLAYER_H
#include <QWidget>
#include "libavcodec/avcodec.h"
#include "libavformat/avformat.h"
#include "libswscale/swscale.h"
class VideoPlayer : public QWidget
{
Q_OBJECT
public:
explicit VideoPlayer(QWidget *parent = 0);
~VideoPlayer();
bool openFile(QString fileName);
void update();
private:
AVFormatContext *formatCtx;
AVCodecContext *codecCtx;
AVCodec *codec;
AVFrame *frame;
AVPicture picture;
int videoStream;
struct SwsContext *swsCtx;
QTimer *timer;
QImage image;
void free();
};
#endif // VIDEOPLAYER_H
```
5. videoplayer.cpp
```
#include "videoplayer.h"
#include <QTimer>
#include <QDebug>
VideoPlayer::VideoPlayer(QWidget *parent) :
QWidget(parent),
formatCtx(NULL),
codecCtx(NULL),
codec(NULL),
frame(NULL),
swsCtx(NULL),
timer(NULL)
{
}
VideoPlayer::~VideoPlayer()
{
free();
}
bool VideoPlayer::openFile(QString fileName)
{
free();
formatCtx = avformat_alloc_context();
if (avformat_open_input(&formatCtx, fileName.toUtf8().data(), NULL, NULL) != 0)
return false;
if (avformat_find_stream_info(formatCtx, NULL) < 0)
return false;
videoStream = -1;
for (unsigned int i = 0; i < formatCtx->nb_streams; ++i)
{
if (formatCtx->streams[i]->codec->codec_type == AVMEDIA_TYPE_VIDEO)
{
videoStream = i;
break;
}
}
if (videoStream == -1)
return false;
codecCtx = formatCtx->streams[videoStream]->codec;
codec = avcodec_find_decoder(codecCtx->codec_id);
if (codec == NULL)
return false;
if (avcodec_open2(codecCtx, codec, NULL) < 0)
return false;
frame = avcodec_alloc_frame();
avpicture_alloc(&picture, PIX_FMT_RGB24, codecCtx->width, codecCtx->height);
swsCtx = sws_getContext(codecCtx->width, codecCtx->height, codecCtx->pix_fmt,
codecCtx->width, codecCtx->height, PIX_FMT_RGB24,
SWS_BILINEAR, NULL, NULL, NULL);
image = QImage(codecCtx->width, codecCtx->height, QImage::Format_RGB888);
return true;
}
void VideoPlayer::update()
{
if (formatCtx == NULL || codecCtx == NULL || frame == NULL || swsCtx == NULL)
return;
AVPacket packet;
while (av_read_frame(formatCtx, &packet) >= 0)
{
if (packet.stream_index == videoStream)
{
int frameFinished;
avcodec_decode_video2(codecCtx, frame, &frameFinished, &packet);
if (frameFinished)
{
sws_scale(swsCtx, frame->data, frame->linesize, 0, codecCtx->height, picture.data, picture.linesize);
image = QImage(picture.data[0], codecCtx->width, codecCtx->height, QImage::Format_RGB888);
}
}
av_free_packet(&packet);
}
if (!image.isNull())
this->update();
}
void VideoPlayer::free()
{
if (formatCtx)
{
avformat_close_input(&formatCtx);
formatCtx = NULL;
}
if (codecCtx)
{
avcodec_close(codecCtx);
codecCtx = NULL;
}
if (frame)
{
av_free(frame);
frame = NULL;
}
avpicture_free(&picture);
if (swsCtx)
{
sws_freeContext(swsCtx);
swsCtx = NULL;
}
}
```
这个简单的示例代码可以让你通过QT界面显示视频文件。请注意,这个示例代码没有处理音频,只能显示视频内容。如果你想要增加音频,你需要添加更多的代码。
相关推荐
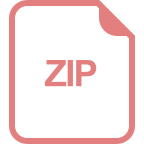
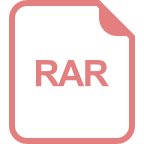















