C# 如何给List、Dictionary扩展一些新的方法
时间: 2023-11-22 20:55:19 浏览: 49
在 C# 中,我们可以使用扩展方法为 List 和 Dictionary 类型添加新的方法。扩展方法是一种特殊的静态方法,它可以像实例方法一样调用,但是它定义在一个静态类中,并且第一个参数用 this 关键字标记,表示该方法是针对该类型的扩展方法。
下面是一个例子,演示如何为 List 类型添加一个新的扩展方法:
```
public static class ListExtensions
{
public static void Print<T>(this List<T> list)
{
foreach (var item in list)
{
Console.WriteLine(item);
}
}
}
```
这个扩展方法名为 Print,它可以输出 List 中的元素。我们将其定义在一个名为 ListExtensions 的静态类中,它的第一个参数是 this List<T>,表示这是一个针对 List<T> 类型的扩展方法。在方法体中,我们使用 foreach 循环遍历 List 中的元素,并输出它们的值。
现在,我们可以在任何 List<T> 实例上调用这个方法,就像调用实例方法一样:
```
List<int> numbers = new List<int> { 1, 2, 3 };
numbers.Print();
```
这将输出:
```
1
2
3
```
同样的方式,我们也可以为 Dictionary 类型添加新的扩展方法。下面是一个例子,演示如何为 Dictionary<string, int> 类型添加一个新的扩展方法:
```
public static class DictionaryExtensions
{
public static void Print(this Dictionary<string, int> dictionary)
{
foreach (var key in dictionary.Keys)
{
Console.WriteLine("{0}: {1}", key, dictionary[key]);
}
}
}
```
这个扩展方法名为 Print,它可以输出 Dictionary 中的键值对。我们将其定义在一个名为 DictionaryExtensions 的静态类中,它的第一个参数是 this Dictionary<string, int>,表示这是一个针对 Dictionary<string, int> 类型的扩展方法。在方法体中,我们使用 foreach 循环遍历 Dictionary 中的键,并输出它们对应的值。
现在,我们可以在任何 Dictionary<string, int> 实例上调用这个方法,就像调用实例方法一样:
```
Dictionary<string, int> scores = new Dictionary<string, int> {
{ "Alice", 99 },
{ "Bob", 85 },
{ "Charlie", 70 }
};
scores.Print();
```
这将输出:
```
Alice: 99
Bob: 85
Charlie: 70
```
通过扩展方法,我们可以为 List、Dictionary 等类型添加新的功能,以便更方便地使用它们。
相关推荐
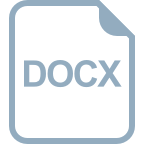
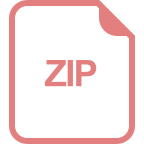
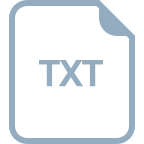











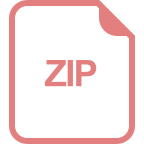
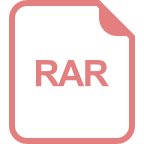
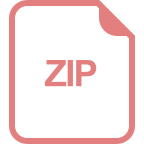
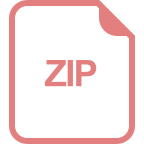