java使用Rijndael加密算法加密的代码
时间: 2023-10-14 09:04:49 浏览: 62
Rijndael是AES加密算法的前身,因此可以使用Java中的AES算法来实现Rijndael加密。以下是一个示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
public class RijndaelEncryption {
private static final String KEY = "1234567890123456"; // 密钥,必须为16位
public static byte[] encrypt(byte[] input) throws Exception {
SecretKeySpec keySpec = new SecretKeySpec(KEY.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, keySpec);
return cipher.doFinal(input);
}
public static byte[] decrypt(byte[] input) throws Exception {
SecretKeySpec keySpec = new SecretKeySpec(KEY.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.DECRYPT_MODE, keySpec);
return cipher.doFinal(input);
}
}
```
使用方法:
```java
byte[] input = "Hello, World!".getBytes();
byte[] encrypted = RijndaelEncryption.encrypt(input); // 加密
byte[] decrypted = RijndaelEncryption.decrypt(encrypted); // 解密
String result = new String(decrypted);
System.out.println(result); // 输出: Hello, World!
```
需要注意的是,密钥必须为16位。如需使用其他长度的密钥,需要使用其他填充方式。
相关推荐
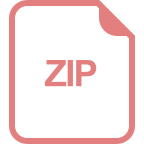














