使用pytorch实现tts的模型
时间: 2023-12-09 11:06:48 浏览: 36
TTS(Text-to-Speech)是一种将文本转化为语音的技术。在PyTorch中,可以使用深度学习模型来实现TTS。以下是一些实现TTS的步骤:
1. 数据预处理:将文本转化为数字序列,并提取声学特征。
2. 搭建模型:使用深度学习模型来学习文本和声学特征之间的映射关系。常用的模型包括Seq2Seq、Tacotron等。
3. 训练模型:使用大量的带有对应语音的文本数据来训练模型。
4. 合成语音:使用训练好的模型,将文本转化为声学特征,并将其转化为语音。
以下是一个基于Tacotron模型的TTS实现示例(假设已经完成了数据预处理):
```python
import torch
import torch.nn as nn
import numpy as np
# 定义Tacotron模型
class Tacotron(nn.Module):
def __init__(self, num_chars, embedding_dim, num_freq, num_hidden):
super(Tacotron, self).__init__()
self.embedding = nn.Embedding(num_chars, embedding_dim)
self.encoder = nn.LSTM(input_size=embedding_dim, hidden_size=num_hidden, batch_first=True)
self.decoder = nn.LSTM(input_size=num_freq, hidden_size=num_hidden, batch_first=True)
self.attention = nn.Linear(2*num_hidden, num_chars)
self.proj = nn.Linear(num_hidden, num_freq)
self.postnet = nn.Sequential(
nn.Conv1d(in_channels=num_freq, out_channels=512, kernel_size=5, padding=2),
nn.BatchNorm1d(512),
nn.ReLU(),
nn.Conv1d(in_channels=512, out_channels=512, kernel_size=5, padding=2),
nn.BatchNorm1d(512),
nn.ReLU(),
nn.Conv1d(in_channels=512, out_channels=num_freq, kernel_size=5, padding=2)
)
def forward(self, input_text, input_spec):
# 编码器
embedded = self.embedding(input_text)
encoder_out, (h_n, c_n) = self.encoder(embedded)
# 解码器
decoder_out, _ = self.decoder(input_spec, (h_n, c_n))
# 注意力
attention_weights = self.attention(torch.cat((decoder_out, encoder_out), dim=-1))
attention_weights = torch.softmax(attention_weights, dim=1)
context_vector = torch.bmm(attention_weights.transpose(1, 2), encoder_out)
# 投影
proj_out = self.proj(decoder_out + context_vector)
# 后处理网络
postnet_out = self.postnet(proj_out.transpose(1, 2)).transpose(1, 2)
# 输出
output_spec = proj_out + postnet_out
output_audio = griffin_lim(output_spec)
return output_spec, output_audio
# 定义损失函数
def TacotronLoss(output_spec, target_spec):
# 计算频谱误差
spec_loss = nn.MSELoss()(output_spec, target_spec)
# 计算声学特征误差
audio_loss = nn.L1Loss()(griffin_lim(output_spec), griffin_lim(target_spec))
# 计算总损失
loss = spec_loss + audio_loss
return loss
# 定义Griffin-Lim算法(用于将声学特征转化为语音)
def griffin_lim(spectrogram):
X_best = torch.zeros(spectrogram.size(0), 1, spectrogram.size(2))
for i in range(30):
X_t = X_best
X_best = spectrogram * torch.exp(1j*angle(torch.stft(X_t.squeeze(1), n_fft=2048, hop_length=256, win_length=2048, window=torch.hann_window(2048).cuda(), center=False)))
X_best = torch.istft(X_best, n_fft=2048, hop_length=256, win_length=2048, window=torch.hann_window(2048).cuda(), center=False)
X_best = X_best.unsqueeze(1)
return X_best.squeeze(1)
# 训练模型
model = Tacotron(num_chars=5000, embedding_dim=256, num_freq=80, num_hidden=512)
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
for epoch in range(100):
for input_text, input_spec, target_spec in train_loader:
output_spec, output_audio = model(input_text, input_spec)
loss = TacotronLoss(output_spec, target_spec)
optimizer.zero_grad()
loss.backward()
optimizer.step()
```
注:上述代码仅作为示例,实际应用中需要根据具体情况进行修改。
相关推荐
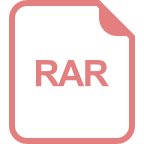
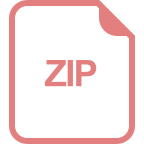
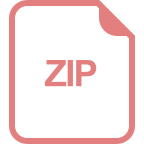
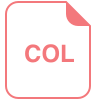
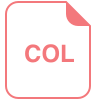
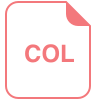
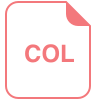
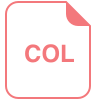










