使用PYTHON怎么把EXCEL文件通过企业微信的机器人发出
时间: 2024-03-07 09:53:48 浏览: 269
可以使用 Python 中的 `requests` 库通过企业微信机器人发送文件。具体步骤如下:
1. 获取企业微信机器人的 Webhook 地址:在企业微信管理后台中,创建一个机器人,获取机器人的 Webhook 地址。将 Webhook 地址保存在一个变量中,例如:
```python
webhook_url = 'https://qyapi.weixin.qq.com/cgi-bin/webhook/send?key=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
```
2. 指定要发送的文件路径:使用 Python 中的 `os` 库指定要发送的文件的路径。
```python
import os
file_path = os.path.join(os.getcwd(), 'example.xlsx') # 假设文件在当前目录下
```
3. 读取文件内容:使用 Python 中的 `open()` 方法打开文件,并使用 `read()` 方法读取文件内容。将文件内容保存在一个变量中,例如:
```python
with open(file_path, 'rb') as f:
file_content = f.read()
```
4. 使用 `requests` 库发送文件:使用 `requests.post()` 方法向机器人的 Webhook 地址发送一个 POST 请求,将文件作为请求的正文发送。
```python
import requests
response = requests.post(webhook_url, data=file_content)
```
在上述代码中,`data` 参数指定了请求的正文,即要发送的文件内容。由于企业微信机器人要求发送的文件必须是 base64 编码后的格式,因此需要在发送前将文件内容进行 base64 编码。可以使用 Python 中的 `base64` 库来进行编码,例如:
```python
import base64
file_content_base64 = base64.b64encode(file_content)
response = requests.post(webhook_url, data=file_content_base64)
```
在发送请求时,还需要在请求头中指定 `Content-Type` 参数为 `application/json`,并在请求正文中指定要发送的文件的文件名和文件类型,例如:
```python
headers = {'Content-Type': 'application/json'}
data = {
'msgtype': 'file',
'file': {
'base64': file_content_base64.decode(),
'filename': 'example.xlsx',
'md5': hashlib.md5(file_content).hexdigest(),
'fileext': 'xlsx'
}
}
response = requests.post(webhook_url, headers=headers, json=data)
```
在上述代码中,`msgtype` 参数指定要发送的消息类型为文件,`file` 参数指定要发送的文件的相关信息,包括 base64 编码后的文件内容、文件名、文件 MD5 值和文件扩展名。
注意,使用企业微信机器人发送文件需要保证机器人账号已经加入了要发送的群聊或个人的联系人列表中。
阅读全文
相关推荐
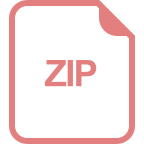


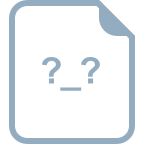
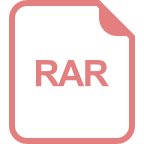
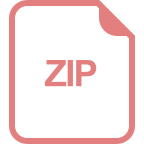
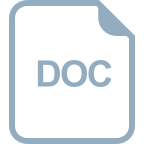

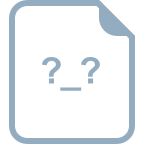
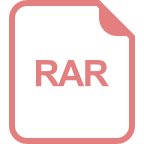
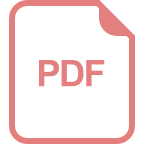
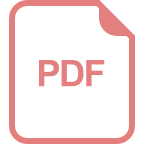
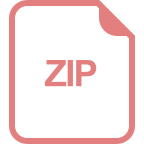
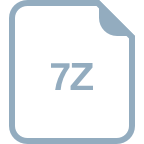
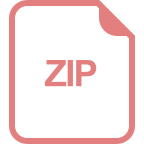
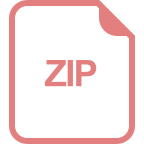