分模块设计一个学生信息库系统。该系统包含学生基本信息的建立和输出、计算学生平均成绩、按照学生的平均成绩排序以及查询、修改学生的成绩等功能。用C语言代码示例。
时间: 2024-03-05 19:47:41 浏览: 26
好的,下面是一个简单的学生信息库系统的分模块设计和C语言代码示例:
1. 建立学生基本信息模块
该模块用于输入学生的基本信息,包括学生姓名、学号、性别、年龄等。
```c
#include <stdio.h>
#include <string.h>
#define MAX_STUDENT_NUM 100 // 最大学生数量
struct student {
char name[20];
int id;
char gender;
int age;
};
struct student students[MAX_STUDENT_NUM]; // 定义学生数组
int student_num = 0; // 当前学生数量
void add_student() {
struct student new_student;
printf("请输入学生姓名:");
scanf("%s", new_student.name);
printf("请输入学号:");
scanf("%d", &new_student.id);
printf("请输入性别(男/女):");
scanf(" %c", &new_student.gender);
printf("请输入年龄:");
scanf("%d", &new_student.age);
students[student_num++] = new_student;
}
```
2. 计算学生成绩模块
该模块用于计算学生的平均成绩。
```c
struct score {
int math;
int english;
int physics;
};
struct score scores[MAX_STUDENT_NUM]; // 定义成绩数组
void add_score() {
int id;
printf("请输入学号:");
scanf("%d", &id);
int index = -1;
for (int i = 0; i < student_num; i++) {
if (students[i].id == id) {
index = i;
break;
}
}
if (index == -1) {
printf("未找到该学生\n");
return;
}
struct score new_score;
printf("请输入数学成绩:");
scanf("%d", &new_score.math);
printf("请输入英语成绩:");
scanf("%d", &new_score.english);
printf("请输入物理成绩:");
scanf("%d", &new_score.physics);
scores[index] = new_score;
}
double get_average_score(int index) {
double sum = scores[index].math + scores[index].english + scores[index].physics;
return sum / 3;
}
```
3. 排序和查询模块
该模块用于按照学生的平均成绩排序并查询学生信息。
```c
void sort_students_by_score() {
for (int i = 0; i < student_num - 1; i++) {
for (int j = i + 1; j < student_num; j++) {
double score1 = get_average_score(i);
double score2 = get_average_score(j);
if (score1 < score2) {
struct student temp_student = students[i];
students[i] = students[j];
students[j] = temp_student;
struct score temp_score = scores[i];
scores[i] = scores[j];
scores[j] = temp_score;
}
}
}
}
void query_student_info() {
int id;
printf("请输入学号:");
scanf("%d", &id);
int index = -1;
for (int i = 0; i < student_num; i++) {
if (students[i].id == id) {
index = i;
break;
}
}
if (index == -1) {
printf("未找到该学生\n");
return;
}
printf("姓名:%s\n", students[index].name);
printf("学号:%d\n", students[index].id);
printf("性别:%c\n", students[index].gender);
printf("年龄:%d\n", students[index].age);
printf("数学成绩:%d\n", scores[index].math);
printf("英语成绩:%d\n", scores[index].english);
printf("物理成绩:%d\n", scores[index].physics);
}
```
4. 修改学生成绩模块
该模块用于修改学生的成绩。
```c
void modify_score() {
int id;
printf("请输入学号:");
scanf("%d", &id);
int index = -1;
for (int i = 0; i < student_num; i++) {
if (students[i].id == id) {
index = i;
break;
}
}
if (index == -1) {
printf("未找到该学生\n");
return;
}
printf("请输入数学成绩:");
scanf("%d", &scores[index].math);
printf("请输入英语成绩:");
scanf("%d", &scores[index].english);
printf("请输入物理成绩:");
scanf("%d", &scores[index].physics);
}
```
完整代码示例:
相关推荐
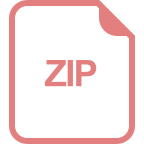

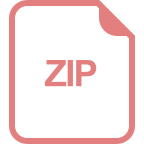
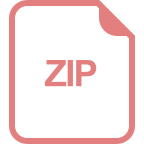
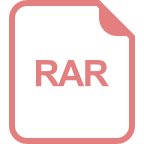
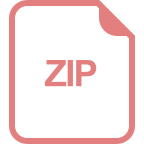
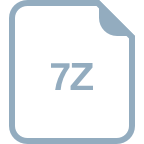
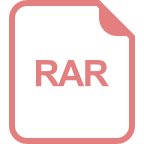
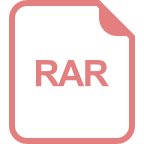
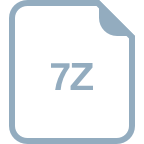
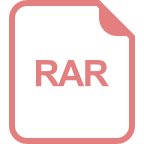
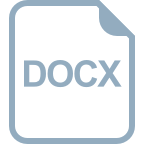
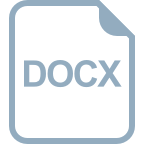
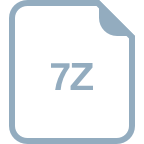