String topLevel = 'top variable'; void main() { var insideMain = 'insideMain variable'; void myFunction() { var insideFunction = 'insideFunction variable'; void nestedFunction() { var insideNestedFunction = 'insideNestedFunction variable'; print('$topLevel'); print('$insideMain'); print('$insideFunction'); print('$insideNestedFunction'); } nestedFunction(); } myFunction(); }
时间: 2024-03-05 14:48:04 浏览: 56
这段代码定义了一个字符串类型的顶层变量 `topLevel`,并赋值为 `'top variable'`。接着定义了一个 `main` 函数,其中声明了一个字符串类型的局部变量 `insideMain`,并赋值为 `'insideMain variable'`。然后定义了一个名为 `myFunction` 的函数,其中声明了一个字符串类型的局部变量 `insideFunction`,并赋值为 `'insideFunction variable'`。在 `myFunction` 函数内部,还定义了一个名为 `nestedFunction` 的函数,其中声明了一个字符串类型的局部变量 `insideNestedFunction`,并赋值为 `'insideNestedFunction variable'`。在 `nestedFunction` 函数内部,分别输出了四个变量的值,其中包括了顶层变量、`main` 函数中的局部变量、`myFunction` 函数中的局部变量,以及 `nestedFunction` 函数中的局部变量。
运行这段代码将会输出如下信息:
```
top variable
insideMain variable
insideFunction variable
insideNestedFunction variable
```
可以看到,这段代码演示了嵌套函数和变量作用域的概念。在嵌套函数中,可以访问外层函数和全局作用域中声明的变量。在这个例子中,`nestedFunction` 函数可以访问所有外层函数和全局作用域中声明的变量,因此可以输出它们的值。
相关问题
如何解决无法将函数类型 '(status: string, code: string) => Promise<void>' 赋值给类型 '($event?: undefined) => any',
在JavaScript中,当你试图将一个特定类型的函数赋值给一个更通用的函数类型时,可能会遇到这种类型不匹配的问题。你提到的函数类型 `(status: string, code: string) => Promise<void>` 指定了接收两个字符串参数并返回一个Promise的情况,而 `($event?: undefined) => any` 则是一个可选参数的回调函数,可以返回任意类型。
为了解决这个问题,你可以做如下几种尝试:
1. **显式类型断言** (Type Assertion): 如果你能确定传入的函数确实符合预期,可以在赋值时加上一个类型断言,告诉 TypeScript 你确信这个函数就是你想的类型。例如:
```typescript
let myFunction: ($event?: undefined) => any = (status, code) => Promise.resolve(); // 类似于此
```
2. **使用接口或类型保护** (Type Guard): 创建一个新的接口,让函数满足这个接口的要求,然后在需要的地方检查它。如果函数符合该接口,则可以安全地赋值。
```typescript
interface MyHandler {
(status: string, code: string): Promise<void>;
}
function isMyHandler(func: unknown): func is MyHandler {
return typeof func === 'function' && typeof func === (status: string, code: string) => void;
}
const myFunction = isMyHandler(someFunction) ? someFunction : null; // 如果someFunction是正确的类型
```
3. **修改目标函数类型**: 如果有可能,考虑调整接受的函数类型,让它能够包容更多的可能性。例如,如果 `$event` 不一定需要,你可以去掉它。
4. **封装函数**: 把原始函数包装到一个新的函数中,使其适应目标类型。
```typescript
function wrapFunction(original: (status: string, code: string) => Promise<void>): ($event?: undefined) => any {
return function(...args) { original(args[0], args[1]); };
}
const wrappedFunction = wrapFunction(myFunction);
```
import java. 1o. * public class MyClass 1 public static void main (String argvl) MyClass mob = new MyClass 0; mob. myFunction ( ); public void myFunction ( ) try i FileReader dis = new FileReader (Hello. txt ); BufferedReader br=new BufferedReader (dis) ; String str=br. readLine); while (str. length () >0) I System. out. printIn (str); Str=br. readLine; catch (FileNotFoundException fne) 1 System. out. printIn("No such file found"); catch (IOException ioe) { finally System. out. printIn("*Ш§T"); 程
这段代码存在一些语法错误,我来帮你修正一下:
```java
import java.io.*;
public class MyClass {
public static void main(String[] argvl) {
MyClass mob = new MyClass();
mob.myFunction();
}
public void myFunction() {
try {
FileReader dis = new FileReader("Hello.txt");
BufferedReader br = new BufferedReader(dis);
String str = br.readLine();
while (str != null && str.length() > 0) {
System.out.println(str);
str = br.readLine();
}
br.close();
dis.close();
} catch (FileNotFoundException fne) {
System.out.println("No such file found");
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
System.out.println("*程序结束");
}
}
}
```
在上述代码中,我们使用 Java IO 的 FileReader 和 BufferedReader 类来读取文件内容。在 myFunction 方法中,我们首先创建 FileReader 和 BufferedReader 对象,然后使用 readLine 方法逐行读取文件内容,直到读取到 null 表示文件结束。在读取文件过程中,我们使用 while 循环不断读取并输出文件内容。最后,我们使用 close 方法关闭 BufferedReader 和 FileReader 对象。如果出现异常,我们使用 try-catch-finally 语句块来处理异常,并在 finally 块中输出一条程序结束的信息。
阅读全文
相关推荐
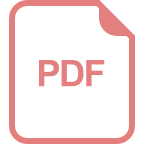
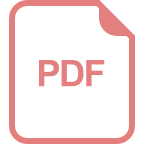
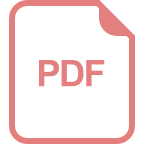
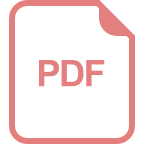
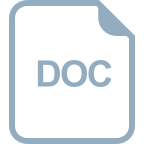










