请用C++实现上述问题,注意每个数的大小是随机的,大于等于1小于等于10000,注意运算优先级
时间: 2024-03-23 21:36:02 浏览: 55
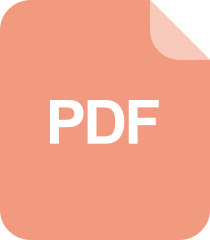
C语言/C++如何生成随机数

好的,下面是一个使用 C++ 实现的代码:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <cmath>
using namespace std;
int randInt(int minVal, int maxVal) {
return minVal + rand() % (maxVal - minVal + 1);
}
int calc(int a, int b, char op) {
if (op == '+') {
return a + b;
} else if (op == '-') {
return a - b;
} else if (op == '*') {
return a * b;
} else if (op == '/') {
return a / b;
}
return 0;
}
int main() {
srand(time(NULL)); // 初始化随机数种子
int a = randInt(1, 10000);
int b = randInt(1, 10000);
int c = randInt(1, 10000);
int d = randInt(1, 10000);
int e = randInt(1, 10000);
int maxVal = 0;
char ops[3] = {'+', '-', '*', '/'};
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
for (int k = 0; k < 4; k++) {
int val = 0;
if (i == 0) {
val = calc(a, b, '*');
val = calc(val, c, '*');
} else if (i == 1) {
val = calc(b, c, '*');
val = calc(a, val, '+');
val = calc(val, d, '+');
} else if (i == 2) {
val = calc(b, c, '/');
val = calc(a, val, '+');
val = calc(val, d, '+');
} else if (i == 3) {
val = calc(a, b, '*');
val = calc(val, c, '/');
val = calc(val, d, '+');
}
val = calc(val, e, '-');
if (val > maxVal) {
maxVal = val;
}
}
}
}
cout << a << " " << b << " " << c << " " << d << " " << e << endl;
cout << "Max value: " << maxVal << endl;
return 0;
}
```
这个程序首先使用 `randInt()` 函数生成五个随机数,并使用三重循环枚举符号的组合情况,计算出每种组合的结果,最终选取其中的最大值输出。
在 `calc()` 函数中,根据符号进行相应的计算,其中 `/` 表示向下取整。
阅读全文
相关推荐
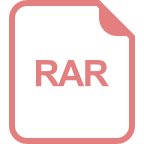
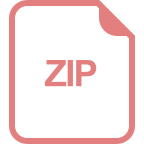
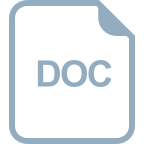
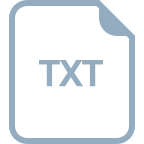
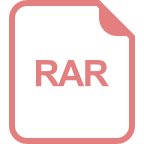
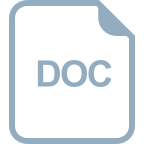
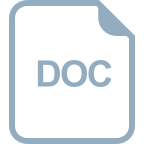
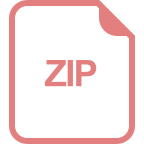
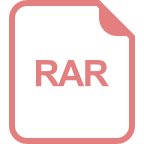
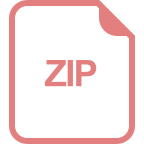
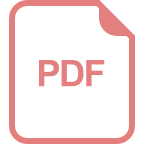
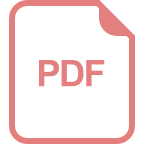