10.由键盘任意输入5个学生的姓名、学号、以及每一个学生的4门功课的成绩,分别计算他们的总分和均分,并将此表按总分的高低排序。写出c语言程序
时间: 2023-06-02 12:04:58 浏览: 79
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STU_NUM 5
#define MAX_NAME_LEN 20
typedef struct {
char name[MAX_NAME_LEN];
int id;
int score[4];
int total_score;
float avg_score;
} Student;
void input_students(Student students[]);
void calculate_score(Student students[]);
void sort_students(Student students[]);
void print_students(Student students[]);
int main() {
Student students[MAX_STU_NUM];
input_students(students);
calculate_score(students);
sort_students(students);
print_students(students);
return 0;
}
// 输入学生信息
void input_students(Student students[]) {
for (int i = 0; i < MAX_STU_NUM; i++) {
printf("请输入第%d个学生的姓名、学号和4门课的成绩:\n", i + 1);
scanf("%s %d %d %d %d %d", students[i].name, &(students[i].id),
&(students[i].score[0]), &(students[i].score[1]), &(students[i].score[2]), &(students[i].score[3]));
}
}
// 计算总分和平均分
void calculate_score(Student students[]) {
for (int i = 0; i < MAX_STU_NUM; i++) {
students[i].total_score = 0;
for (int j = 0; j < 4; j++) {
students[i].total_score += students[i].score[j];
}
students[i].avg_score = (float) students[i].total_score / 4;
}
}
// 按总分排序
void sort_students(Student students[]) {
for (int i = 0; i < MAX_STU_NUM - 1; i++) {
for (int j = 0; j < MAX_STU_NUM - i - 1; j++) {
if (students[j].total_score < students[j + 1].total_score) {
Student temp = students[j];
students[j] = students[j + 1];
students[j + 1] = temp;
}
}
}
}
// 输出学生信息
void print_students(Student students[]) {
printf("按总分从高到低排名如下:\n");
printf("姓名\t学号\t科目1\t科目2\t科目3\t科目4\t总分\t平均分\n");
for (int i = 0; i < MAX_STU_NUM; i++) {
printf("%s\t%d\t%d\t%d\t%d\t%d\t%d\t%.1f\n", students[i].name, students[i].id,
students[i].score[0], students[i].score[1], students[i].score[2], students[i].score[3],
students[i].total_score, students[i].avg_score);
}
}
```
相关推荐
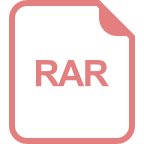








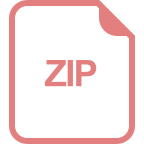
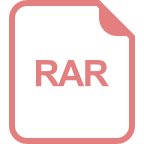
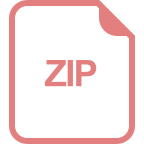
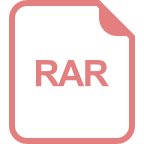
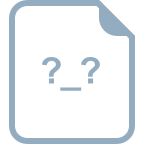
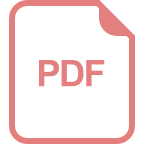