C++编写一个学生选课系统的用户类注册程序,要求用户账号密码数据可以保存到文本文档中,并且将整个程序封装为用户流类
时间: 2024-03-01 11:55:30 浏览: 102
好的,我可以帮你编写这个程序。首先,我们需要定义一个用户类,用来存储用户账号和密码:
```cpp
#include <string>
#include <fstream>
class User {
public:
User(const std::string& username, const std::string& password) :
m_username(username), m_password(password) {}
const std::string& getUsername() const {
return m_username;
}
const std::string& getPassword() const {
return m_password;
}
bool operator==(const User& other) const {
return m_username == other.m_username &&
m_password == other.m_password;
}
private:
std::string m_username;
std::string m_password;
};
```
接下来,我们需要定义一个用户流类,用来封装用户类和文件读写操作:
```cpp
class UserStream {
public:
UserStream(const std::string& filename) : m_filename(filename) {}
bool registerUser(const User& user) {
// 检查用户名是否已经存在
for (const auto& u : m_users) {
if (u == user) {
return false;
}
}
// 添加用户
m_users.push_back(user);
// 保存到文件
std::ofstream ofs(m_filename, std::ios::app);
if (!ofs) {
return false;
}
ofs << user.getUsername() << ' ' << user.getPassword() << std::endl;
return true;
}
bool loginUser(const std::string& username, const std::string& password) const {
// 检查用户名和密码是否匹配
for (const auto& u : m_users) {
if (u.getUsername() == username && u.getPassword() == password) {
return true;
}
}
return false;
}
private:
std::string m_filename;
std::vector<User> m_users;
};
```
现在,我们可以在主函数中使用用户流类来注册和登录用户:
```cpp
int main() {
UserStream userStream("users.txt");
// 注册用户
User user1("user1", "password1");
if (userStream.registerUser(user1)) {
std::cout << "User registered" << std::endl;
}
else {
std::cout << "Failed to register user" << std::endl;
}
// 登录用户
if (userStream.loginUser("user1", "password1")) {
std::cout << "User logged in" << std::endl;
}
else {
std::cout << "Failed to log in user" << std::endl;
}
return 0;
}
```
这个程序会将用户账号和密码保存到名为“users.txt”的文本文档中。如果需要保存到其他文件,只需要修改构造函数中的文件名即可。
阅读全文
相关推荐

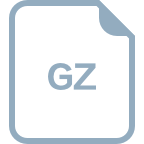




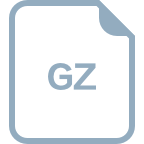

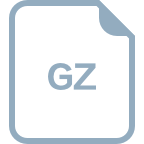