java数字连连消小游戏代码
时间: 2023-08-13 13:15:43 浏览: 107
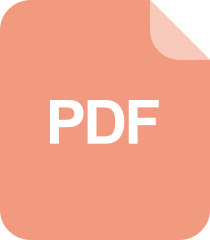
Java实现数字连连消
以下是一个简单的 Java 数字连连消小游戏代码示例:
```java
import java.awt.*;
import java.awt.event.*;
import java.util.*;
import javax.swing.*;
public class NumberMatchingGame extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 400;
private static final int HEIGHT = 400;
private static final int ROWS = 4;
private static final int COLUMNS = 4;
private static final int DELAY = 1000;
private static final int FONT_SIZE = 30;
private static final int MAX_NUMBER = ROWS * COLUMNS / 2;
private static final Random rand = new Random();
private Timer timer;
private int[][] board;
private JButton[][] buttons;
private int firstRow, firstColumn, secondRow, secondColumn;
private int count;
private int score;
private JLabel countLabel, scoreLabel;
public NumberMatchingGame() {
super("Number Matching Game");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(WIDTH, HEIGHT);
setResizable(false);
setLocationRelativeTo(null);
setLayout(new BorderLayout());
JPanel boardPanel = new JPanel(new GridLayout(ROWS, COLUMNS));
buttons = new JButton[ROWS][COLUMNS];
board = new int[ROWS][COLUMNS];
for (int r = 0; r < ROWS; r++) {
for (int c = 0; c < COLUMNS; c++) {
buttons[r][c] = new JButton();
buttons[r][c].setFont(new Font("Arial", Font.BOLD, FONT_SIZE));
buttons[r][c].addActionListener(this);
boardPanel.add(buttons[r][c]);
}
}
add(boardPanel, BorderLayout.CENTER);
JPanel infoPanel = new JPanel(new GridLayout(1, 2));
countLabel = new JLabel("Count: 0", JLabel.CENTER);
countLabel.setFont(new Font("Arial", Font.PLAIN, FONT_SIZE));
infoPanel.add(countLabel);
scoreLabel = new JLabel("Score: 0", JLabel.CENTER);
scoreLabel.setFont(new Font("Arial", Font.PLAIN, FONT_SIZE));
infoPanel.add(scoreLabel);
add(infoPanel, BorderLayout.SOUTH);
timer = new Timer(DELAY, this);
setVisible(true);
initGame();
}
private void initGame() {
count = 0;
score = 0;
countLabel.setText("Count: " + count);
scoreLabel.setText("Score: " + score);
int[] numbers = new int[MAX_NUMBER];
for (int i = 0; i < MAX_NUMBER; i++) {
numbers[i] = i + 1;
}
shuffle(numbers);
int index = 0;
for (int r = 0; r < ROWS; r++) {
for (int c = 0; c < COLUMNS; c++) {
board[r][c] = numbers[index];
buttons[r][c].setText(Integer.toString(board[r][c]));
buttons[r][c].setEnabled(true);
index++;
}
}
timer.start();
}
private void shuffle(int[] array) {
for (int i = array.length - 1; i > 0; i--) {
int j = rand.nextInt(i + 1);
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
public void actionPerformed(ActionEvent e) {
Object source = e.getSource();
if (source == timer) {
timer.stop();
hideNumbers();
} else {
for (int r = 0; r < ROWS; r++) {
for (int c = 0; c < COLUMNS; c++) {
if (source == buttons[r][c]) {
if (count == 0) {
firstRow = r;
firstColumn = c;
buttons[r][c].setEnabled(false);
count++;
} else if (count == 1) {
secondRow = r;
secondColumn = c;
buttons[r][c].setEnabled(false);
count++;
if (board[firstRow][firstColumn] == board[secondRow][secondColumn]) {
score++;
scoreLabel.setText("Score: " + score);
if (score == MAX_NUMBER) {
JOptionPane.showMessageDialog(this, "You win!");
initGame();
}
} else {
timer.start();
}
} else {
buttons[firstRow][firstColumn].setEnabled(true);
buttons[secondRow][secondColumn].setEnabled(true);
firstRow = r;
firstColumn = c;
buttons[r][c].setEnabled(false);
count = 1;
}
}
}
}
}
}
private void hideNumbers() {
buttons[firstRow][firstColumn].setEnabled(true);
buttons[secondRow][secondColumn].setEnabled(true);
buttons[firstRow][firstColumn].setText("");
buttons[secondRow][secondColumn].setText("");
count = 0;
}
public static void main(String[] args) {
new NumberMatchingGame();
}
}
```
这个小游戏的规则很简单:用户需要翻开数字牌,每次翻开两张数字牌,如果两张数字相同,则得分,继续翻下一对数字牌,直到翻完全部数字牌。如果翻开的两张数字不相同,则需要在一定时间内记住数字的位置,然后数字牌会再次翻转回去。用户需要根据记忆找到两张相同的数字牌,才能继续游戏。如果用户成功翻开所有数字牌,则游戏胜利。
阅读全文
相关推荐
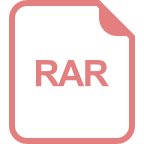
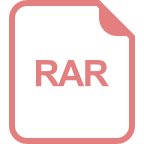
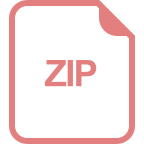
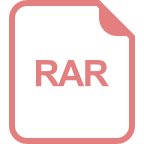
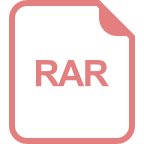
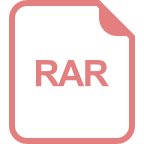
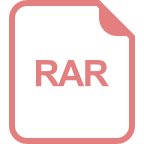
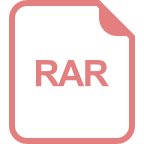
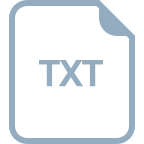
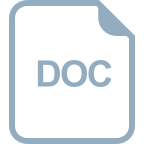
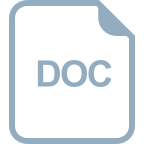
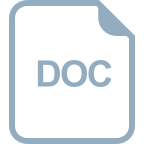
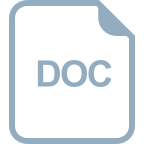
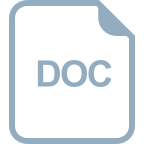
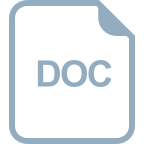
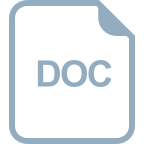