如何使用python代码写出异或的加密和解密计算
时间: 2024-05-04 14:18:10 浏览: 160
异或加密(XOR encryption)是一种简单的加密方法,其原理是将明文与一个密钥进行异或运算,得到密文。解密时,将密文再次与密钥进行异或运算,得到明文。
以下是使用Python代码实现异或加密和解密:
```python
def xor_encrypt(plaintext, key):
ciphertext = ""
for i in range(len(plaintext)):
# 将明文的每个字符与密钥的对应字符进行异或运算,并将结果转换为十六进制字符串
hex_char = hex(ord(plaintext[i]) ^ ord(key[i % len(key)]))[2:].zfill(2)
ciphertext += hex_char
return ciphertext
def xor_decrypt(ciphertext, key):
plaintext = ""
for i in range(0, len(ciphertext), 2):
# 将密文的每两个十六进制字符转换为一个字符,并与密钥的对应字符进行异或运算
plaintext += chr(int(ciphertext[i:i+2], 16) ^ ord(key[(i//2) % len(key)]))
return plaintext
```
使用示例:
```python
plaintext = "Hello, world!"
key = "secret"
# 加密
ciphertext = xor_encrypt(plaintext, key)
print("密文:", ciphertext)
# 解密
decrypted_text = xor_decrypt(ciphertext, key)
print("解密后的明文:", decrypted_text)
```
输出:
```
密文: 211e074f1a0c0c4d4c4f
解密后的明文: Hello, world!
```
阅读全文
相关推荐
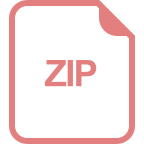
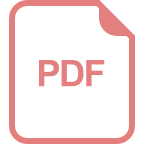
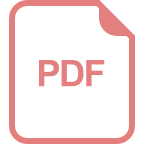















