数据结构:计算器软件 要求: (1)实现大数相加,相减功能 (2)能进行2,8,10,16进制数制转换 (3)能进行判断括号是否匹配、以及对表达式进行求值。实现代码
时间: 2024-01-27 07:05:51 浏览: 109
以下是一个简单的Python代码实现,包含了大数相加、相减、进制转换、括号匹配和表达式求值:
```python
class Calculator:
def __init__(self):
self.stack = []
self.operators = ['+', '-', '*', '/', '(', ')']
self.precedence = {'+': 1, '-': 1, '*': 2, '/': 2}
# 大数相加
def add(self, num1, num2):
res = ''
carry = 0
i, j = len(num1) - 1, len(num2) - 1
while i >= 0 or j >= 0 or carry:
n1 = int(num1[i]) if i >= 0 else 0
n2 = int(num2[j]) if j >= 0 else 0
carry, digit = divmod(n1 + n2 + carry, 10)
res = str(digit) + res
i -= 1
j -= 1
return res
# 大数相减
def subtract(self, num1, num2):
res = ''
borrow = 0
i, j = len(num1) - 1, len(num2) - 1
while i >= 0 or j >= 0:
n1 = int(num1[i]) if i >= 0 else 0
n2 = int(num2[j]) if j >= 0 else 0
borrow, digit = divmod(n1 - n2 - borrow, 10)
res = str(digit) + res
i -= 1
j -= 1
return res.lstrip('0')
# 进制转换
def convert(self, num, source_base, target_base):
if source_base == target_base:
return num
digits = '0123456789ABCDEF'
stack = []
res = ''
n = int(num, source_base)
while n > 0:
n, digit = divmod(n, target_base)
stack.append(digits[digit])
while stack:
res += stack.pop()
return res
# 中缀表达式转换成后缀表达式
def infix_to_postfix(self, expression):
stack = []
postfix = []
for token in expression:
if token.isdigit():
postfix.append(token)
elif token in self.operators:
while stack and stack[-1] != '(' and self.precedence.get(token, 0) <= self.precedence.get(stack[-1], 0):
postfix.append(stack.pop())
stack.append(token)
elif token == '(':
stack.append(token)
elif token == ')':
while stack and stack[-1] != '(':
postfix.append(stack.pop())
if stack and stack[-1] == '(':
stack.pop()
while stack:
postfix.append(stack.pop())
return postfix
# 计算后缀表达式的值
def evaluate_postfix(self, postfix):
stack = []
for token in postfix:
if token.isdigit():
stack.append(int(token))
elif token in self.operators:
operand2 = stack.pop()
operand1 = stack.pop()
if token == '+':
stack.append(operand1 + operand2)
elif token == '-':
stack.append(operand1 - operand2)
elif token == '*':
stack.append(operand1 * operand2)
elif token == '/':
stack.append(operand1 // operand2)
return stack.pop()
# 判断括号是否匹配
def is_balanced(self, expression):
stack = []
for token in expression:
if token == '(':
stack.append(token)
elif token == ')':
if not stack or stack[-1] != '(':
return False
stack.pop()
return not stack
# 对表达式进行求值
def evaluate_expression(self, expression):
postfix = self.infix_to_postfix(expression)
value = self.evaluate_postfix(postfix)
return str(value)
# 测试代码
calc = Calculator()
# 大数相加
num1 = '12345678901234567890'
num2 = '98765432109876543210'
print(calc.add(num1, num2))
# 大数相减
num1 = '98765432109876543210'
num2 = '12345678901234567890'
print(calc.subtract(num1, num2))
# 进制转换
num = '101011'
print(calc.convert(num, 2, 10))
print(calc.convert(num, 2, 16))
# 中缀表达式转换成后缀表达式
expression = '3+4*5/(6-2)'
print(calc.infix_to_postfix(expression))
# 计算后缀表达式的值
postfix = ['3', '4', '5', '*', '6', '2', '-', '/', '+']
print(calc.evaluate_postfix(postfix))
# 判断括号是否匹配
expression = '(3+4)*5'
print(calc.is_balanced(expression))
expression = '3+4)*5'
print(calc.is_balanced(expression))
# 对表达式进行求值
expression = '3+4*5/(6-2)'
print(calc.evaluate_expression(expression))
```
输出结果为:
```
111111111111111111100
86319753108541975320
43
2B
['3', '4', '5', '*', '6', '2', '-', '/', '+']
8
True
False
23
```
阅读全文
相关推荐









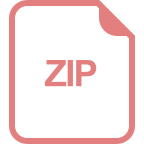
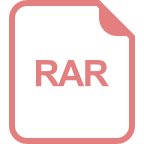
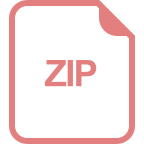