在PYQT中显示检测标签(0-9)进行打印输出文件,VS2022进行读取文件展示在WIN界面上
时间: 2023-05-25 12:03:29 浏览: 139
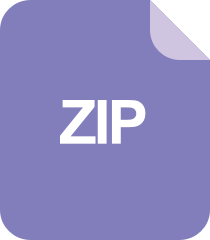
基于python-contrib-opencv+dlib+pyqt5的视频读取人脸录入检测项目源码.zip
以下是一个简单的示例程序,展示了如何在PYQT中显示和打印检测标签,并将输出文件保存到本地。然后,使用VS2022读取保存的文件,并在WIN界面上展示文件内容。
在PYQT中显示和打印检测标签的代码如下:
```python
from PyQt5.QtWidgets import *
from PyQt5.QtGui import *
from PyQt5.QtPrintSupport import *
import sys
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建菜单栏
menu_bar = self.menuBar()
file_menu = menu_bar.addMenu("File")
# 添加"Print"菜单项
print_action = QAction("Print", self)
print_action.triggered.connect(self.printer)
file_menu.addAction(print_action)
# 创建并设置中心窗口
self.widget = QWidget()
self.setCentralWidget(self.widget)
# 创建布局
layout = QVBoxLayout()
self.widget.setLayout(layout)
# 添加检测标签
self.labels = []
for i in range(10):
label = QLabel(str(i))
self.labels.append(label)
layout.addWidget(label)
def printer(self):
# 打印输出文件
dialog = QFileDialog(self)
dialog.setDefaultSuffix("txt")
file_path, _ = dialog.getSaveFileName(self, "Save File", "", "Text Files (*.txt)")
if file_path:
with open(file_path, "w") as f:
for i in range(10):
f.write(str(i) + "\n")
printer = QPrinter()
printer.setOutputFormat(QPrinter.NativeFormat)
printer.setPaperSize(QPrinter.A4)
printer.setFullPage(True)
painter = QPainter()
painter.begin(printer)
for label in self.labels:
label.render(painter)
painter.end()
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
保存输出文件后,在VS2022中读取并展示文件内容的代码如下:
```c++
#include <fstream>
#include <string>
#include <iostream>
#include <Windows.h>
int main()
{
// 读取文件
std::ifstream file("C:/output.txt");
std::string content((std::istreambuf_iterator<char>(file)), std::istreambuf_iterator<char>());
// 在WIN界面上展示文件内容
WCHAR* wtext = new WCHAR[content.size() + 1];
MultiByteToWideChar(CP_ACP, 0, content.c_str(), -1, wtext, content.size() + 1);
wtext[content.size()] = '\0';
MessageBox(NULL, wtext, L"Output File Content", MB_OK);
delete[] wtext;
return 0;
}
```
注意:需要将文件路径`C:/output.txt`替换为实际保存的文件路径。
阅读全文
相关推荐
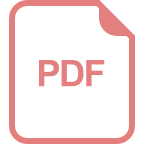
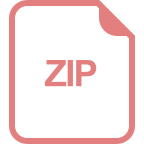
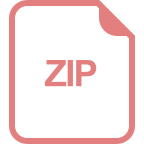
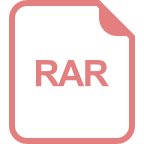
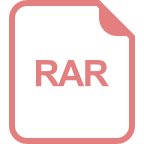
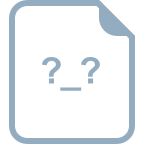
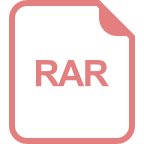
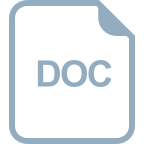
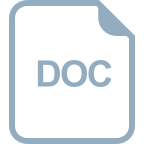
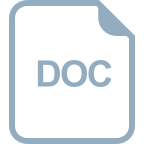






