用Python画一个喜羊羊的代码
时间: 2023-08-31 08:27:24 浏览: 274
### 回答1:
我们可以使用 Python 的第三方库 `turtle` 来画一个喜羊羊。首先,你需要安装 `turtle` 库:
```
pip install turtle
```
然后,你可以使用以下代码来画一个喜羊羊:
```
import turtle
t = turtle.Turtle()
# 画头
t.circle(50)
# 画身体
t.penup()
t.goto(0, -50)
t.pendown()
t.circle(100, 180)
# 画腿
t.penup()
t.goto(-50, -200)
t.pendown()
t.circle(50, 180)
t.penup()
t.goto(50, -200)
t.pendown()
t.circle(50, 180)
# 画眼睛
t.penup()
t.goto(-20, 50)
t.pendown()
t.circle(10)
t.penup()
t.goto(20, 50)
t.pendown()
t.circle(10)
# 画嘴巴
t.penup()
t.goto(-20, 20)
t.pendown()
t.goto(20, 20)
t.penup()
t.goto(0, 20)
t.pendown()
t.goto(0, 10)
# 隐藏画笔
t.penup()
# 让窗口等待关闭
turtle.exitonclick()
```
运行这段代码,你就可以看到一个画有喜羊羊的窗口弹出来了!
### 回答2:
要用Python画一个喜羊羊的代码,可以使用turtle库来实现。
首先,导入turtle库并创建一个画布,设置画布大小和背景颜色。
```python
import turtle
# 创建画布
window = turtle.Screen()
window.title("喜羊羊")
window.bgcolor("white")
window.setup(width=800, height=600)
```
接下来,创建一个绘图工具turtle,并设置画笔的颜色和尺寸。
```python
# 创建绘图工具
pen = turtle.Turtle()
pen.color("black")
pen.pensize(3)
```
然后,用turtle的绘制方法来画出喜羊羊的形状。
```python
# 画羊的头部
pen.circle(50)
# 画左耳朵
pen.goto(-35, 120)
pen.setheading(-40)
pen.circle(60, 80)
pen.setheading(40)
pen.circle(60, 80)
# 画右耳朵
pen.goto(35, 120)
pen.setheading(140)
pen.circle(-60, 80)
pen.setheading(-40)
pen.circle(-60, 80)
# 画左眼
pen.goto(-15, 155)
pen.fillcolor("black")
pen.begin_fill()
pen.circle(10)
pen.end_fill()
# 画右眼
pen.goto(15, 155)
pen.fillcolor("black")
pen.begin_fill()
pen.circle(10)
pen.end_fill()
# 画鼻子
pen.goto(0, 130)
pen.setheading(-90)
pen.forward(25)
pen.setheading(0)
pen.circle(25)
# 画嘴巴
pen.goto(-25, 130)
pen.setheading(-60)
pen.circle(30, 120)
# 画身体
pen.goto(0, 50)
pen.setheading(-60)
pen.circle(100, 120)
pen.goto(0, 50)
pen.setheading(60)
pen.circle(-100, 120)
pen.penup()
pen.goto(0, 50)
pen.setheading(60)
pen.circle(-100, 120)
pen.pendown()
# 画腿
pen.goto(0, -50)
pen.setheading(-60)
pen.forward(100)
pen.goto(0, -50)
pen.setheading(60)
pen.forward(100)
# 画尾巴
pen.goto(-25, -50)
pen.setheading(-100)
pen.circle(25, 90)
# 完成绘画
pen.hideturtle()
turtle.done()
```
运行上述代码,就会在画布上画出一个喜羊羊的形状。你可以调整代码中的参数,比如尺寸、颜色等,来得到你喜欢的喜羊羊。
### 回答3:
import turtle
# 设置画布
canvas = turtle.Screen()
canvas.title("喜羊羊")
canvas.bgcolor("white")
# 创建一个小乌龟对象
xYY = turtle.Turtle()
xYY.shape("turtle")
xYY.color("black")
# 绘制头部
xYY.penup()
xYY.goto(0, -100)
xYY.pendown()
xYY.circle(100)
# 绘制眼睛
xYY.penup()
xYY.goto(-40, 30)
xYY.pendown()
xYY.fillcolor("white")
xYY.begin_fill()
xYY.circle(20)
xYY.end_fill()
xYY.penup()
xYY.goto(40, 30)
xYY.pendown()
xYY.begin_fill()
xYY.circle(20)
xYY.end_fill()
# 绘制嘴巴
xYY.penup()
xYY.goto(-40, 0)
xYY.pendown()
xYY.setheading(60)
xYY.circle(40, 120)
# 绘制身体
xYY.penup()
xYY.goto(-150, -50)
xYY.pendown()
xYY.setheading(0)
xYY.fillcolor("brown")
xYY.begin_fill()
xYY.forward(300)
xYY.left(90)
for _ in range(2):
xYY.forward(100)
xYY.right(90)
xYY.forward(200)
xYY.right(90)
xYY.forward(100)
xYY.end_fill()
# 绘制腿
xYY.penup()
xYY.goto(-150, -150)
xYY.pendown()
xYY.setheading(0)
xYY.fillcolor("brown")
xYY.begin_fill()
for _ in range(2):
xYY.forward(40)
xYY.right(90)
xYY.forward(100)
xYY.right(90)
xYY.forward(40)
xYY.end_fill()
# 绘制脚
xYY.penup()
xYY.goto(-110, -250)
xYY.pendown()
xYY.setheading(0)
xYY.fillcolor("brown")
xYY.begin_fill()
for _ in range(2):
xYY.forward(80)
xYY.right(90)
xYY.forward(40)
xYY.right(90)
xYY.forward(80)
xYY.end_fill()
# 绘制尾巴
xYY.penup()
xYY.goto(150, -50)
xYY.pendown()
xYY.setheading(135)
xYY.pensize(15)
xYY.forward(200)
xYY.backward(200)
# 隐藏画笔
xYY.hideturtle()
# 结束绘图
turtle.done()
相关推荐
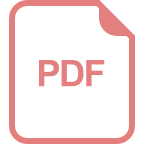
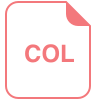
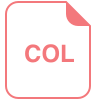
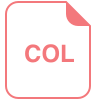
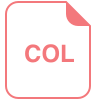
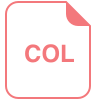
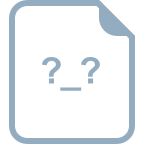
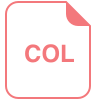
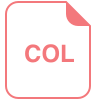
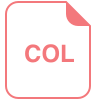
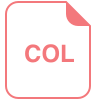
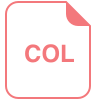
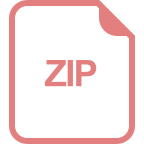