Implement the hash table (with chaining) data structure. Your table should be able to grow and shrink based on how many items are there. contain at least the following methods: * __init__: initialize the data structure * hash: implement a hash function that is chosen from a universal hash family * search: search for a key in the table, return True if found, False otherwise * insert: insert a key into the table if it is not already in there * delete: delete a key from the table * size: return the number of buckets in your hash table
时间: 2024-02-14 09:02:52 浏览: 70
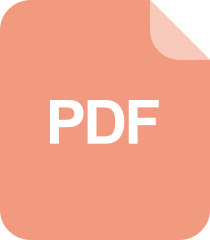
Swift.Data.Structure.and.Algorithms.2016.11.pdf
Sure, I can help you with that. Here's a possible implementation of a hash table with chaining:
```python
import random
class HashTable:
def __init__(self, initial_size=10, load_factor=0.7):
self.buckets = [[] for _ in range(initial_size)]
self.load_factor = load_factor
self.size = 0
def hash(self, key):
# A simple universal hash function using random parameters
a = random.randint(1, 10**9)
b = random.randint(0, 10**9)
p = 10**9 + 7
return (a * hash(key) + b) % p % len(self.buckets)
def search(self, key):
bucket = self.buckets[self.hash(key)]
for k, v in bucket:
if k == key:
return True
return False
def insert(self, key, value=None):
if self.size / len(self.buckets) >= self.load_factor:
self._resize(2 * len(self.buckets))
bucket = self.buckets[self.hash(key)]
for i, (k, v) in enumerate(bucket):
if k == key:
bucket[i] = (key, value)
return
bucket.append((key, value))
self.size += 1
def delete(self, key):
bucket = self.buckets[self.hash(key)]
for i, (k, v) in enumerate(bucket):
if k == key:
del bucket[i]
self.size -= 1
if self.size / len(self.buckets) <= 1 - self.load_factor:
self._resize(len(self.buckets) // 2)
return
raise KeyError(key)
def _resize(self, new_size):
new_buckets = [[] for _ in range(new_size)]
for bucket in self.buckets:
for k, v in bucket:
new_buckets[self.hash(k)].append((k, v))
self.buckets = new_buckets
def __len__(self):
return self.size
```
This implementation uses a list of buckets, where each bucket is a list of key-value pairs. The hash function is a simple universal hash function that uses random parameters to avoid collisions. The load factor determines when the table should be resized (either doubled or halved) to maintain a reasonable level of occupancy. The `search`, `insert`, `delete`, and `size` methods are straightforward operations on the buckets. The `__len__` method returns the number of key-value pairs in the table.
Note that this implementation doesn't handle collisions beyond chaining, and doesn't support efficient iteration over all key-value pairs in the table. There are many possible improvements and variations depending on the specific use case and performance requirements.
阅读全文
相关推荐
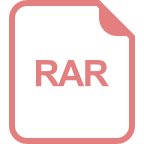
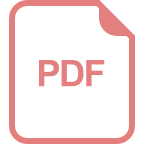
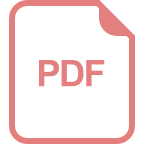
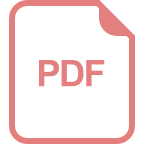
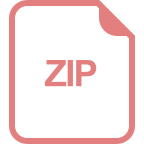
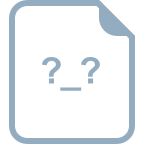
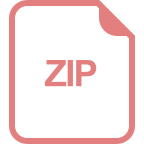
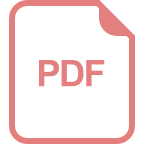
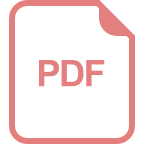
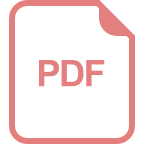
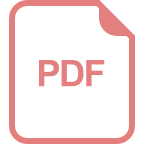
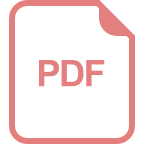
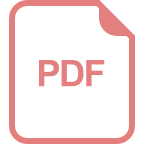