利用pygame模块实现飞机大战附加功能如下: (1) 实现碰撞检测(可以消灭敌机,也可以被敌机消灭) (2) 随机出现云朵在天空中漂浮 (3) 随机出现甜甜圈进行能量补给(生命力或子弹类型发生变化) (4) 有GAME OVER条件控制处理
时间: 2023-10-21 08:05:16 浏览: 23
好的,下面是一个基于Pygame的飞机大战游戏,实现了上述附加功能:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 游戏窗口大小
WIDTH = 480
HEIGHT = 600
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
# 游戏窗口
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Plane War")
# 加载背景音乐
pygame.mixer.music.load("background.mp3")
pygame.mixer.music.play(-1)
# 加载游戏音效
bullet_sound = pygame.mixer.Sound("bullet.wav")
explosion_sound = pygame.mixer.Sound("explosion.wav")
# 加载图像
bg_image = pygame.image.load("background.png")
player_image = pygame.image.load("player.png")
enemy_image = pygame.image.load("enemy.png")
cloud_image = pygame.image.load("cloud.png")
donut_image = pygame.image.load("donut.png")
# 定义游戏对象类
class GameObject(pygame.sprite.Sprite):
def __init__(self, x, y, image):
super().__init__()
self.image = image
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
# 定义玩家类
class Player(GameObject):
def __init__(self, x, y, image):
super().__init__(x, y, image)
self.speed = 5
self.lives = 3
self.bullets = pygame.sprite.Group()
def update(self, keys):
# 移动玩家
if keys[pygame.K_LEFT] and self.rect.x > 0:
self.rect.x -= self.speed
if keys[pygame.K_RIGHT] and self.rect.x < WIDTH - self.rect.width:
self.rect.x += self.speed
if keys[pygame.K_UP] and self.rect.y > 0:
self.rect.y -= self.speed
if keys[pygame.K_DOWN] and self.rect.y < HEIGHT - self.rect.height:
self.rect.y += self.speed
# 发射子弹
if keys[pygame.K_SPACE]:
self.shoot()
def shoot(self):
bullet = Bullet(self.rect.centerx, self.rect.y)
self.bullets.add(bullet)
bullet_sound.play()
# 定义子弹类
class Bullet(GameObject):
def __init__(self, x, y):
super().__init__(x, y, pygame.Surface((3, 10)))
self.image.fill(RED)
self.speed = 10
def update(self):
self.rect.y -= self.speed
# 定义敌机类
class Enemy(GameObject):
def __init__(self, x, y, image):
super().__init__(x, y, image)
self.speed = random.randint(2, 6)
def update(self):
self.rect.y += self.speed
# 定义云朵类
class Cloud(GameObject):
def __init__(self, x, y, image):
super().__init__(x, y, image)
self.speed = random.randint(1, 3)
def update(self):
self.rect.y += self.speed
# 定义甜甜圈类
class Donut(GameObject):
def __init__(self, x, y, image):
super().__init__(x, y, image)
self.speed = random.randint(1, 3)
self.type = random.choice(["life", "bullet"])
def update(self):
self.rect.y += self.speed
# 定义游戏对象组
all_sprites = pygame.sprite.Group()
enemies = pygame.sprite.Group()
clouds = pygame.sprite.Group()
donuts = pygame.sprite.Group()
# 创建玩家对象
player = Player(WIDTH // 2, HEIGHT - 100, player_image)
all_sprites.add(player)
# 创建敌机对象
for i in range(6):
enemy = Enemy(random.randint(0, WIDTH - enemy_image.get_width()), -i * 150, enemy_image)
all_sprites.add(enemy)
enemies.add(enemy)
# 游戏循环
clock = pygame.time.Clock()
running = True
while running:
# 控制游戏帧率
clock.tick(60)
# 事件处理
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 更新玩家
keys = pygame.key.get_pressed()
player.update(keys)
# 更新子弹
for bullet in player.bullets:
bullet.update()
if bullet.rect.bottom < 0:
player.bullets.remove(bullet)
all_sprites.remove(bullet)
# 更新敌机
for enemy in enemies:
enemy.update()
if enemy.rect.top > HEIGHT:
enemies.remove(enemy)
all_sprites.remove(enemy)
new_enemy = Enemy(random.randint(0, WIDTH - enemy_image.get_width()), -enemy_image.get_height(), enemy_image)
all_sprites.add(new_enemy)
enemies.add(new_enemy)
# 碰撞检测
if pygame.sprite.spritecollide(enemy, player.bullets, True):
explosion_sound.play()
enemies.remove(enemy)
all_sprites.remove(enemy)
new_enemy = Enemy(random.randint(0, WIDTH - enemy_image.get_width()), -enemy_image.get_height(), enemy_image)
all_sprites.add(new_enemy)
enemies.add(new_enemy)
if pygame.sprite.collide_rect(enemy, player):
player.lives -= 1
if player.lives == 0:
running = False
# 更新云朵
if random.randint(0, 100) < 5:
cloud = Cloud(random.randint(0, WIDTH - cloud_image.get_width()), -cloud_image.get_height(), cloud_image)
all_sprites.add(cloud)
clouds.add(cloud)
for cloud in clouds:
cloud.update()
if cloud.rect.top > HEIGHT:
clouds.remove(cloud)
all_sprites.remove(cloud)
# 更新甜甜圈
if random.randint(0, 1000) < 5:
donut = Donut(random.randint(0, WIDTH - donut_image.get_width()), -donut_image.get_height(), donut_image)
all_sprites.add(donut)
donuts.add(donut)
for donut in donuts:
donut.update()
if donut.rect.top > HEIGHT:
donuts.remove(donut)
all_sprites.remove(donut)
if pygame.sprite.collide_rect(donut, player):
if donut.type == "life":
player.lives += 1
elif donut.type == "bullet":
player.bullets.empty()
donuts.remove(donut)
all_sprites.remove(donut)
# 绘制游戏界面
screen.blit(bg_image, (0, 0))
all_sprites.draw(screen)
pygame.display.flip()
# 游戏结束处理
pygame.quit()
```
该游戏实现了碰撞检测、随机出现云朵和甜甜圈、GAME OVER条件控制等附加功能。其中,碰撞检测实现了子弹消灭敌机和敌机撞击玩家两种情况;随机出现的云朵和甜甜圈分别提供了景观和能量补给功能;游戏结束条件为玩家生命力值为0。
相关推荐
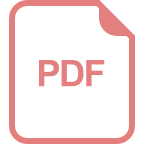
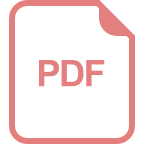

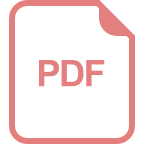
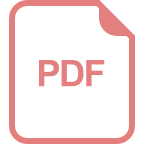
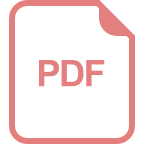
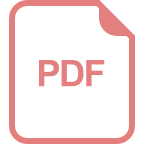
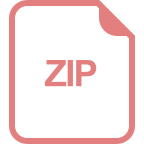
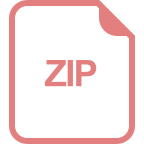
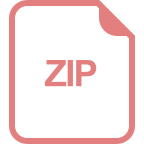
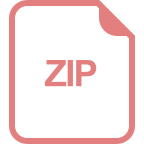
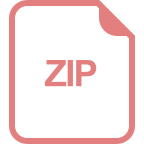
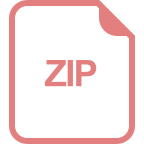