用有一链式结构,定义如下 : struct stu{ char name[20]; int no; struct stu *next; }; 创建一个函数create(),实现无头结点的链表,有m个结点,函数的返回值为链表的头指针。 函数接口定义: 在这里描述函数接口。例如: struct stu * create() 在这里解释接口参数。在函数中输入m,及m行数据,完成链表的创建。 裁判测试程序样例: 在这里给出函数被调用进行测试的例子。例如: #include <stdio.h> #include <stdlib.h> struct stu{ char name[20]; int no; struct stu *next;}; struct stu * create(); void show(struct stu *p){ while(p){ printf("%s %d\n",p->name,p->no); p=p->next; } } int main() { struct stu *p=NULL; p=create(); show(p); return 0; } /* 请在这里填写答案 */
时间: 2024-03-19 10:41:29 浏览: 75
struct stu *create() {
int m;
scanf("%d", &m);
struct stu *head = NULL, *tail = NULL;
for(int i = 0; i < m; i++) {
struct stu *node = (struct stu*)malloc(sizeof(struct stu));
scanf("%s%d", node->name, &node->no);
node->next = NULL;
if(head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
相关问题
有一链式结构,定义如下 : struct stu{ char name[20]; int no; struct stu *next;
这是一个链式结构体,其中包含了三个成员:name、no 和 next。其中,name 是一个长度为 20 的 char 数组,用来存储学生姓名;no 是一个整型变量,用来存储学号;next 是一个指向下一个结构体的指针,用来构建链表。
这个链式结构体可以用来表示一个学生信息,每个结构体代表一个学生,通过 next 指针可以将多个学生信息串联起来形成一个链表。在链表中,每个结构体代表一个节点,next 指针指向下一个节点,最后一个节点的 next 指针为 NULL,表示链表的末尾。
有一链式结构,定义如下 : struct stu{ char name[20]; int no; struct stu *next; }; 创建一个函数create(),实现无头结点的链表,有m个结点,函数的返回值为链表的头指针。
下面是一个实现该功能的create()函数的示例代码:
```
struct stu* create(int m){
struct stu *head = NULL, *p = NULL;
int i;
for(i = 0; i < m; i++){
p = (struct stu*)malloc(sizeof(struct stu));
printf("请输入第%d个学生的姓名和学号:", i+1);
scanf("%s%d", p->name, &(p->no));
p->next = head;
head = p;
}
return head;
}
```
该函数接受一个整数m作为参数,表示链表中的结点个数。在函数内部,首先定义了一个头指针head和一个结点指针p。然后通过循环不断读入学生信息,每读入一个学生信息就动态分配一个结点并将其插入链表头部。最后返回头指针head。需要注意的是,由于是无头结点的链表,所以在插入第一个结点时,头指针head应该初始化为NULL。
阅读全文
相关推荐
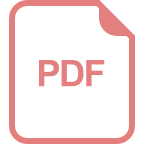
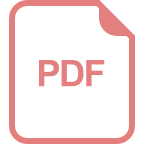
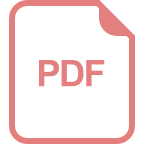




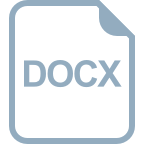
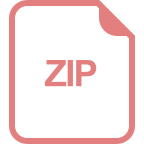
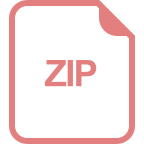
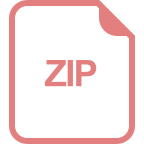
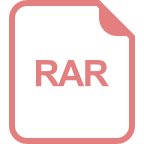
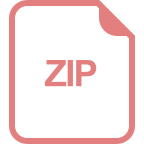
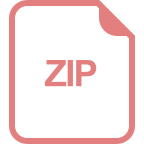