#include <linux/module.h> #include <linux/inetdevice.h> #include <linux/netdevice.h> #include <linux/types.h> #include <linux/if_ether.h> #include <linux/string.h> #include <linux/kernel.h> #include <linux/init.h> #include <linux/in.h> #include <linux/inet.h> #include <linux/socket.h> #include <linux/netfilter.h> #include <linux/netfilter_ipv4.h> #include <net/netfilter/nf_conntrack.h> #include <linux/netfilter/nf_conntrack_tuple_common.h> #include <linux/netfilter/nf_conntrack_common.h> #include <linux/version.h> #include <net/sock.h> #include <net/route.h> #include <net/flow.h>和#include <linux/module.h> #include <linux/inetdevice.h> #include <linux/netdevice.h> #include <linux/types.h> #include <linux/if_ether.h> #include <linux/string.h> #include <linux/kernel.h> #include <linux/init.h> #include <linux/in.h> #include <linux/inet.h> #include <linux/socket.h> #include <linux/version.h> #include <linux/netfilter.h> #include <net/sock.h> #include <net/route.h> #include <net/flow.h> #include <net/ip.h>对应的在vpp中的头文件
时间: 2023-08-14 15:06:41 浏览: 52
在vpp中,这些头文件对应的可能是:
- <vnet/vnet.h>:包含了许多与网络相关的数据类型、函数和宏定义。
- <vnet/ip/ip.h>:包含了IP协议相关的数据类型、函数和宏定义。
- <vnet/tcp/tcp.h>:包含了TCP协议相关的数据类型、函数和宏定义。
- <vnet/udp/udp.h>:包含了UDP协议相关的数据类型、函数和宏定义。
- <vnet/ethernet/ethernet.h>:包含了以太网协议相关的数据类型、函数和宏定义。
- <vnet/ip/ip4.h>:包含了IPv4协议相关的数据类型、函数和宏定义。
- <vnet/ip/ip6.h>:包含了IPv6协议相关的数据类型、函数和宏定义。
- <vnet/tcp/tcp_header.h>:包含了TCP报文头相关的数据类型、函数和宏定义。
- <vnet/udp/udp_header.h>:包含了UDP报文头相关的数据类型、函数和宏定义。
相关问题
linux mac802.11,linux wireless 基础知识 MAC80211 CFG80211(示例代码)
Linux中的MAC80211和CFG80211是用于无线网络的子系统。它们提供了一组API,使开发人员能够在Linux内核中实现无线网络设备驱动程序。
MAC80211是一个实现IEEE 802.11标准的软件模块,它负责管理Linux内核中的无线网络设备。它为网络设备提供了一组接口,使它们能够与其他网络设备进行通信。MAC80211还负责处理无线帧和管理无线网络的连接。
CFG80211是一个用于配置802.11设备的API。它负责管理无线网络设备的配置,例如频率、信道和加密设置等。它还提供了一组接口,使用户空间应用程序能够与无线网络设备进行通信。
示例代码:
以下代码展示了如何使用CFG80211 API在Linux内核中配置无线网络设备。
```
#include <linux/module.h>
#include <linux/kernel.h>
#include <linux/netdevice.h>
#include <linux/wireless.h>
#include <net/cfg80211.h>
static struct cfg80211_ops my_cfg_ops = {
.change_beacon = NULL,
};
static struct cfg80211_device my_cfg_device = {
.ops = &my_cfg_ops,
};
static int __init my_init(void)
{
int ret;
struct wireless_dev *wdev;
wdev = kzalloc(sizeof(*wdev), GFP_KERNEL);
if (!wdev)
return -ENOMEM;
wdev->wiphy = wiphy_new(&my_cfg_ops, sizeof(*wdev));
if (!wdev->wiphy) {
kfree(wdev);
return -ENOMEM;
}
wdev->wiphy->privid++;
wdev->wiphy->dev.parent = NULL;
wdev->wiphy->dev.release = NULL;
wdev->wiphy->dev.groups = NULL;
wdev->wiphy->dev.dma_mask = NULL;
wdev->wiphy->dev.coherent_dma_mask = ~0;
ret = wiphy_register(wdev->wiphy);
if (ret) {
wiphy_free(wdev->wiphy);
kfree(wdev);
return ret;
}
wdev->wiphy->dev.parent = wiphy_dev(wdev->wiphy);
wdev->netdev = alloc_netdev_mqs(sizeof(struct net_device *), "my_dev",
NET_NAME_UNKNOWN, ether_setup,
1, 1);
if (!wdev->netdev) {
wiphy_unregister(wdev->wiphy);
wiphy_free(wdev->wiphy);
kfree(wdev);
return -ENOMEM;
}
wdev->wiphy->privid++;
wdev->netdev->ieee80211_ptr = wdev;
wdev->netdev->ieee80211_ptr->iftype = NL80211_IFTYPE_STATION;
wdev->netdev->ieee80211_ptr->flags |= IEEE80211_STA_CONNECTION_POLL;
ret = register_netdev(wdev->netdev);
if (ret) {
free_netdev(wdev->netdev);
wiphy_unregister(wdev->wiphy);
wiphy_free(wdev->wiphy);
kfree(wdev);
return ret;
}
my_cfg_device.wiphy = wdev->wiphy;
ret = cfg80211_register_device(&my_cfg_device);
if (ret) {
unregister_netdev(wdev->netdev);
free_netdev(wdev->netdev);
wiphy_unregister(wdev->wiphy);
wiphy_free(wdev->wiphy);
kfree(wdev);
return ret;
}
return 0;
}
static void __exit my_exit(void)
{
cfg80211_unregister_device(&my_cfg_device);
unregister_netdev(wdev->netdev);
free_netdev(wdev->netdev);
wiphy_unregister(wdev->wiphy);
wiphy_free(wdev->wiphy);
kfree(wdev);
}
module_init(my_init);
module_exit(my_exit);
MODULE_LICENSE("GPL");
```
这段代码首先创建了一个无线设备和一个无线网络接口。然后,它将无线设备注册到CFG80211子系统中,并将无线网络接口注册到Linux内核中。最后,它将无线设备和无线网络接口添加到一个数据结构中,并将该数据结构注册到CFG80211子系统中。
写一个linux rgmii接口的网络驱动代码
在Linux系统中,rgmii接口的网络驱动代码可以使用如下方式编写:#include <linux/kernel.h>
#include <linux/module.h>
#include <linux/netdevice.h>
#include <linux/phy.h>
#include <linux/mii.h>
#include <linux/if_ether.h>
#include <linux/etherdevice.h>static int rgmii_probe(struct net_device *ndev)
{
struct rgmii_priv *priv;
struct phy_device *phy_dev;
int ret; priv = netdev_priv(ndev); phy_dev = of_phy_connect(ndev, priv->phy_node,
rgmii_adjust_link, 0, priv->interface);
if (!phy_dev) {
dev_err(&ndev->dev, "no PHY found\n");
return -ENODEV;
} priv->phy_dev = phy_dev; ret = register_netdev(ndev);
if (ret) {
dev_err(&ndev->dev, "register_netdev() failed\n");
return ret;
} return 0;
}static const struct net_device_ops rgmii_netdev_ops = {
.ndo_open = rgmii_open,
.ndo_stop = rgmii_stop,
.ndo_start_xmit = rgmii_start_xmit,
.ndo_set_rx_mode = rgmii_set_rx_mode,
.ndo_change_mtu = rgmii_change_mtu,
.ndo_get_stats64 = rgmii_get_stats64,
};static int __init rgmii_init_module(void)
{
return platform_driver_register(&rgmii_driver);
}module_init(rgmii_init_module);static void __exit rgmii_exit_module(void)
{
platform_driver_unregister(&rgmii_driver);
}module_exit(rgmii_exit_module);MODULE_LICENSE("GPL");
MODULE_AUTHOR("John Doe <john.doe@example.com>");
相关推荐
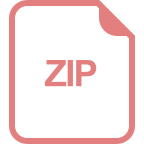
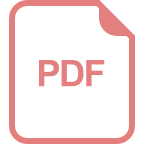










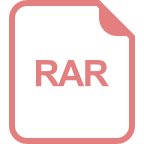
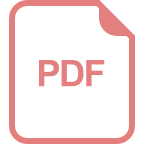
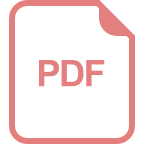