Write comprehensive program in Java which include all the common features of OOP with displaying results of variables using the methods
时间: 2024-04-09 07:28:00 浏览: 126
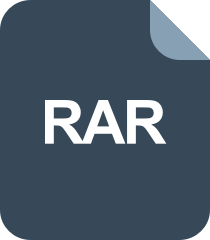
Java_jobs_in_the_soft_questions.rar_The Word
Certainly! I can help you write a comprehensive Java program that includes all the common features of Object-Oriented Programming (OOP) and displays the results of variables using methods. Here's an example program:
```java
public class OOPExample {
// Define a class
public static class Person {
// Define instance variables
private String name;
private int age;
// Define constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Define instance methods
public void sayHello() {
System.out.println("Hello, my name is " + name);
}
public void displayAge() {
System.out.println("I am " + age + " years old");
}
}
// Define another class that inherits from Person
public static class Student extends Person {
private String major;
public Student(String name, int age, String major) {
super(name, age);
this.major = major;
}
public void displayMajor() {
System.out.println("I am majoring in " + major);
}
}
// Main method
public static void main(String[] args) {
// Create an instance of Person and call methods
Person person = new Person("John", 25);
person.sayHello();
person.displayAge();
// Create an instance of Student and call methods
Student student = new Student("Alice", 20, "Computer Science");
student.sayHello();
student.displayAge();
student.displayMajor();
}
}
```
In this program, we define a `Person` class with instance variables `name` and `age`, as well as instance methods `sayHello()` and `displayAge()`. We also define a `Student` class that inherits from `Person` and adds an additional instance variable `major` and a method `displayMajor()`.
In the `main` method, we create an instance of `Person` and call its methods to display the name and age. Then, we create an instance of `Student` and call its methods to display the name, age, and major.
When you run this program, it will output the following:
```
Hello, my name is John
I am 25 years old
Hello, my name is Alice
I am 20 years old
I am majoring in Computer Science
```
This example program demonstrates the common features of OOP in Java, including classes, objects, instance variables, constructors, and instance methods. It also shows how to use methods to display the results of variables.
阅读全文
相关推荐
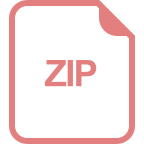
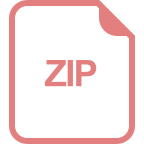















