linux编写程序利用无名管道通信实现命令cat的功能
时间: 2023-09-08 17:12:09 浏览: 48
要实现命令cat的功能,我们可以编写两个程序,一个程序作为父进程,另一个程序作为子进程,通过无名管道进行通信。
以下是父进程的代码:
```
#include <stdlib.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
int main()
{
int fd[2];
pid_t pid;
char buf[1024];
if (pipe(fd) < 0) {
perror("pipe error");
exit(1);
}
if ((pid = fork()) < 0) {
perror("fork error");
exit(1);
} else if (pid > 0) { /* parent process */
close(fd[0]); /* close read end */
while (fgets(buf, sizeof(buf), stdin) != NULL) {
int len = strlen(buf);
if (write(fd[1], buf, len) != len) {
perror("write error");
exit(1);
}
}
close(fd[1]); /* close write end */
wait(NULL);
} else { /* child process */
close(fd[1]); /* close write end */
if (dup2(fd[0], STDIN_FILENO) != STDIN_FILENO) {
perror("dup2 error");
exit(1);
}
close(fd[0]); /* close read end */
execlp("cat", "cat", NULL);
perror("execlp error");
exit(1);
}
return 0;
}
```
父进程从标准输入中读取数据,然后将数据写入到无名管道中,最后关闭写端。子进程从无名管道中读取数据,并通过标准输出将数据输出出来。
编译运行程序:
```
$ gcc pipe_cat.c -o pipe_cat
$ echo "Hello, world!" | ./pipe_cat
Hello, world!
```
这里使用了echo命令将字符串"Hello, world!"写入到标准输入中,并通过管道传递给pipe_cat程序。最后,程序输出了"Hello, world!"。由于子进程是通过execlp函数来执行cat命令的,因此这个程序可以实现cat命令的所有功能。
相关推荐
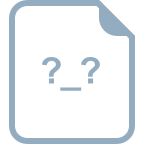














