Main.java:10: error: interface expected here class Oval implements Shape{ ^ Main.java:5: error: Main.Shape is not abstract and does not override abstract method perimeter() in Main.Shape class Shape{ ^ Main.java:6: error: abstract methods cannot have a body abstract double area(){}; ^ Main.java:7: error: abstract methods cannot have a body abstract double perimeter(){}; ^ Main.java:23: error: cannot find symbol return PI*a*b; ^ symbol: variable PI location: class Main.Oval Main.java:26: error: cannot find symbol return 2*PI*b+4*(a-b); //L=2πb+4(a-b) ^ symbol: variable PI location: class Main.Oval 6 errors
时间: 2023-11-27 22:49:49 浏览: 57
这段代码在编译时出现了6个错误,其中有以下几个问题:
1. 在 `class Oval implements Shape` 中,应该实现接口而不是继承类。所以应该改为 `class Oval implements Shape`。
2. 在抽象类中,抽象方法不能有方法体。所以需要去掉 `abstract double area(){};` 和 `abstract double perimeter(){};` 中的花括号。
3. 在 `Oval` 类中,计算面积和周长时出现了错误。在 Java 中,π的值可以使用 `Math.PI` 来表示,而不是直接使用 `PI`。
修改后的代码如下所示:
```
interface Shape {
double area();
double perimeter();
}
class Rectangle implements Shape {
double length;
double width;
Rectangle(double l, double w) {
length = l;
width = w;
}
public double area() {
return length * width;
}
public double perimeter() {
return 2 * (length + width);
}
}
class Oval implements Shape {
double a;
double b;
Oval(double x, double y) {
a = x;
b = y;
}
public double area() {
return Math.PI * a * b;
}
public double perimeter() {
return 2 * Math.PI * b + 4 * (a - b); // L=2πb+4(a-b)
}
}
public class Main {
public static void main(String[] args) {
Shape rect = new Rectangle(5, 10);
Shape oval = new Oval(5, 10);
System.out.println("Rectangle Area: " + rect.area());
System.out.println("Rectangle Perimeter: " + rect.perimeter());
System.out.println("Oval Area: " + oval.area());
System.out.println("Oval Perimeter: " + oval.perimeter());
}
}
```
相关推荐
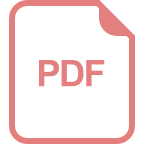
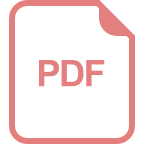
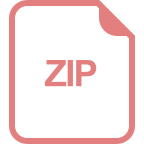














