boost::lockfree::spsc_queue有哪些常用函数
时间: 2023-09-27 18:11:45 浏览: 211
boost::lockfree::spsc_queue有以下常用函数:
1. push():向队列尾部添加一个元素;
2. pop():从队列头部弹出一个元素;
3. front():获取队列头部的元素;
4. empty():判断队列是否为空;
5. size():获取队列中元素的数量;
6. is_lock_free():判断该队列是否无锁(即该队列是否线程安全)。
需要注意的是,boost::lockfree::spsc_queue只能被一个生产者线程和一个消费者线程同时使用。
相关问题
boost::spsc_queue 多线程安全吗
boost::spsc_queue是一个单生产者单消费者队列,它的多线程安全性取决于如何使用。在单生产者单消费者的场景下,只有一个线程会对队列进行操作,因此不需要加锁。但是,在多个生产者或消费者的情况下,为了保证线程安全,需要进行锁保护。
具体来说,如果多个线程同时对队列进行读写操作,那么需要使用线程同步机制(例如互斥锁)来确保多线程访问的安全性。在使用boost::spsc_queue时,需要开发者自己进行多线程安全的实现。
需要注意的是,如果多个线程同时进行写操作,可能会导致数据竞争和不一致的问题,因此需要采取相应的并发控制措施来保证数据的正确性。
请使用C加加实现SPSC
SPSC (Single Producer Single Consumer) 是一种并发编程模型,其中一个线程作为生产者将数据放入队列,另一个线程作为消费者从队列中取出数据。以下是使用 C++11 标准库中的原子操作和条件变量实现的 SPSC 队列。
```c++
#include <atomic>
#include <condition_variable>
#include <mutex>
template <typename T>
class SPSCQueue {
public:
SPSCQueue() : head_(new Node), tail_(head_.load()) {}
~SPSCQueue() {
while (Node* const old_head = head_) {
head_ = old_head->next_;
delete old_head;
}
}
void push(const T& value) {
Node* const new_node = new Node(value);
tail_->next_ = new_node;
tail_ = new_node;
std::unique_lock<std::mutex> lock(mutex_);
++num_items_;
lock.unlock();
cv_.notify_one();
}
bool pop(T& value) {
std::unique_lock<std::mutex> lock(mutex_);
cv_.wait(lock, [this] { return num_items_ > 0; });
Node* const old_head = head_;
head_ = old_head->next_;
value = old_head->value_;
--num_items_;
lock.unlock();
delete old_head;
return true;
}
private:
struct Node {
Node() : next_(nullptr) {}
Node(const T& value) : value_(value), next_(nullptr) {}
T value_;
Node* next_;
};
std::atomic<Node*> head_;
std::atomic<Node*> tail_;
std::atomic<int> num_items_{0};
std::mutex mutex_;
std::condition_variable cv_;
SPSCQueue(const SPSCQueue&) = delete;
SPSCQueue& operator=(const SPSCQueue&) = delete;
};
```
在上述实现中,使用 `std::atomic` 模板定义了原子类型的头尾指针和计数器,保证了多线程情况下对它们的操作是原子的。同时使用 `std::mutex` 和 `std::condition_variable` 实现了线程安全的生产者-消费者模型,其中消费者线程在队列为空时等待,生产者线程在向队列中添加元素时通知消费者线程。
阅读全文
相关推荐
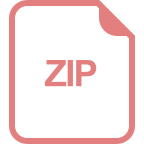
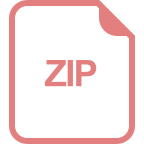







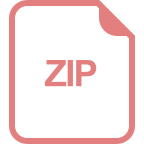
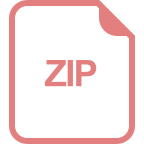
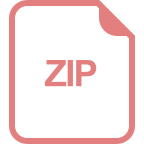
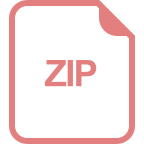
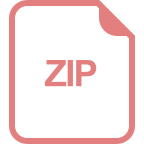
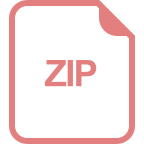
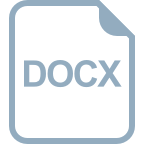