CFG生成树C语言代码
时间: 2023-11-12 11:13:08 浏览: 60
CFG(Context-Free Grammar,上下文无关文法)的生成树可以用递归下降分析法来实现。下面是一个简单的CFG的C语言生成树代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 100
char input_str[MAX_LEN + 1];
int index = 0;
void S();
void A();
void match(char c) {
if (input_str[index] == c) {
index++;
} else {
printf("Error! Expected %c but got %c\n", c, input_str[index]);
exit(1);
}
}
void S() {
printf("S -> ");
if (input_str[index] == 'a') {
match('a');
A();
match('b');
} else {
printf("Error! Expected 'a' but got %c\n", input_str[index]);
exit(1);
}
}
void A() {
printf("A -> ");
if (input_str[index] == 'c') {
match('c');
A();
} else {
printf("A -> ε\n");
}
}
int main() {
printf("Enter a string: ");
fgets(input_str, MAX_LEN, stdin);
input_str[strcspn(input_str, "\n")] = '\0';
S();
if (input_str[index] == '\0') {
printf("Accepted!\n");
} else {
printf("Rejected! Extra characters found.\n");
}
return 0;
}
```
在这个示例中,我们定义了一个简单的CFG,它由两个非终结符 `S` 和 `A` 组成,以及三个终结符 `a`、`b`、`c`。这个CFG的语法规则是:
```
S -> a A b
A -> c A | ε
```
其中 `ε` 表示空产生式。
然后,我们定义了两个函数 `S` 和 `A`,用来生成生成树。这两个函数的实现基于递归下降分析法。我们还定义了一个 `match` 函数,用来匹配输入的字符和 CFG 中定义的字符是否一致。
最后,我们在 `main` 函数中读入输入字符串,并调用 `S` 函数来生成生成树。如果生成树成功并且所有的输入字符都被匹配,那么就输出 `Accepted!`,否则就输出 `Rejected!`。
阅读全文
相关推荐
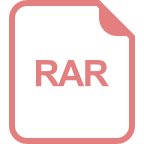
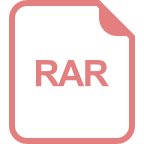
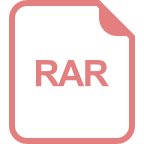
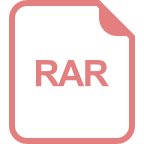
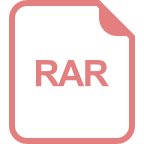
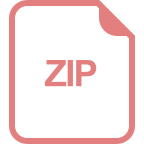
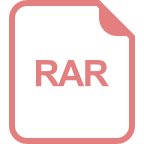
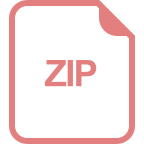
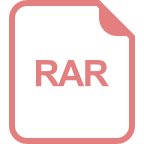
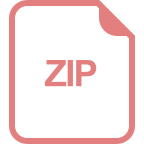
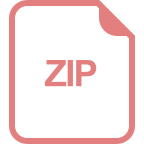
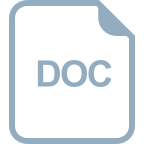
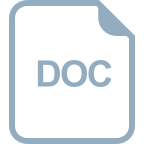
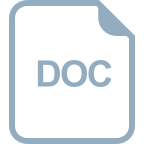
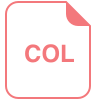
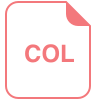