教我用php写一个新年烟花代码
时间: 2023-09-03 21:25:11 浏览: 124
### 回答1:
下面是一个简单的 PHP 烟花代码示例:
```
<?php
for ($i = 1; $i <= 100; $i++) {
$x = rand(1, 100);
$y = rand(1, 100);
echo "<div style='position:absolute;left:".$x."px;top:".$y."px;color:white;'>*</div>";
}
?>
```
这段代码会在页面中随机生成 100 个 "*",模拟烟花效果。
你可以根据自己的需求更改代码,例如更换烟花图案,改变烟花颜色、数量等等。
注意,这只是一个简单的示例,如果要在实际项目中使用,需要进行更多的优化和调整。
### 回答2:
使用PHP编写一个新年烟花代码可以通过以下步骤实现:
首先,需要创建一个HTML页面,代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>新年烟花</title>
<style>
canvas {
background: #000000;
}
</style>
</head>
<body>
<canvas id="fireworks"></canvas>
<script src="fireworks.js"></script>
</body>
</html>
```
然后,需要创建JavaScript文件`fireworks.js`,代码如下:
```javascript
window.onload = function() {
// 创建画布
var canvas = document.getElementById('fireworks');
var ctx = canvas.getContext('2d');
var particles = [];
var MAX_PARTICLES = 100; // 最大粒子数
var hue = 120; // 烟花颜色
// 创建粒子对象
function Particle() {
this.x = canvas.width / 2;
this.y = canvas.height / 2;
this.vx = Math.random() * 6 - 3;
this.vy = Math.random() * -10 - 1;
this.color = 'hsla(' + hue + ', 100%, 50%, 0.8)';
this.size = Math.random() * 2;
}
// 更新粒子位置和速度
Particle.prototype.update = function() {
this.x += this.vx;
this.y += this.vy;
this.vy += 0.1;
this.size -= 0.02;
}
// 绘制粒子
Particle.prototype.draw = function() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.size, 0, Math.PI * 2);
ctx.fillStyle = this.color;
ctx.fill();
}
// 创建粒子爆炸效果
function createParticles() {
if (particles.length < MAX_PARTICLES) {
for (var i = 0; i < 10; i++) {
particles.push(new Particle());
}
}
}
// 绘制烟花效果
function drawFireworks() {
ctx.globalCompositeOperation = 'destination-out';
ctx.fillStyle = 'rgba(0,0,0,0.1)';
ctx.fillRect(0, 0, canvas.width, canvas.height);
ctx.globalCompositeOperation = 'lighter';
for (var i = 0; i < particles.length; i++) {
var p = particles[i];
p.draw();
p.update();
if (p.size <= 0) {
particles.splice(i, 1);
i--;
}
}
requestAnimationFrame(drawFireworks);
}
setInterval(function() {
hue += 2;
}, 50);
function launchFireworks() {
setInterval(function() {
createParticles();
}, 150);
drawFireworks();
}
launchFireworks();
}
```
最后,将以上代码保存为`index.html`和`fireworks.js`文件,并将它们放置在同一个目录下。打开浏览器,并通过访问`index.html`页面,便可以看到绚丽的新年烟花效果。
以上就是使用PHP编写一个新年烟花代码的简单实现。请注意,这只是一个简单的示例,实际上,PHP主要用于处理服务器端的逻辑,与客户端的页面交互一般使用JavaScript实现。
### 回答3:
可以使用PHP编写一个简单的新年烟花代码,以下是一个可能的实现:
```php
<?php
// 创建画布
$image = imagecreatetruecolor(800, 600);
$background = imagecolorallocate($image, 0, 0, 0);
imagefill($image, 0, 0, $background);
// 定义烟花颜色
$colors = [
imagecolorallocate($image, 255, 0, 0),
imagecolorallocate($image, 0, 255, 0),
imagecolorallocate($image, 0, 0, 255),
imagecolorallocate($image, 255, 255, 0),
imagecolorallocate($image, 0, 255, 255),
imagecolorallocate($image, 255, 0, 255)
];
// 生成烟花
function createFirework($x, $y, $color) {
global $image;
$radius = 5;
$particles = 100;
for ($i = 0; $i < $particles; $i++) {
$angle = (pi() * 2 / $particles) * $i;
$distance = rand(1, $radius);
$particleX = $x + cos($angle) * $distance;
$particleY = $y + sin($angle) * $distance;
imagesetpixel($image, $particleX, $particleY, $color);
}
}
// 随机生成烟花
for ($i = 0; $i < 50; $i++) {
$x = rand(50, 750);
$y = rand(50, 550);
$color = $colors[rand(0, count($colors) - 1)];
createFirework($x, $y, $color);
}
// 输出图像
header('Content-Type: image/png');
imagepng($image);
imagedestroy($image);
?>
```
这段代码会创建一个800x600像素的画布,并在其中随机生成50个烟花形状的图案。烟花的颜色会从预定义的颜色中随机选择,并生成具有不同半径的5个部分。最后,将图像输出为PNG格式并销毁画布。
你可以将这段代码保存为`fireworks.php`文件,并通过访问该文件来查看新年烟花效果。注意,你需要在支持PHP的服务器上运行该文件。
相关推荐
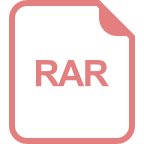
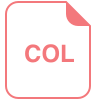
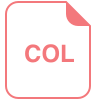
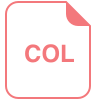
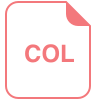
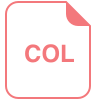









