优化代码#include<bits/stdc++.h> using namespace std; int main(){ int n,m,k; vector<int>v; cin>>n; while(n--){ cin>>m>>k; if(m==1){ v.push_back(k); } if(m==2){ vector<int>::iterator it = find(v.begin(),v.end(),k); if(it!=v.end()) cout<<"True"<<endl; else cout<<"False"<<endl; } } }
时间: 2023-06-18 07:02:03 浏览: 100
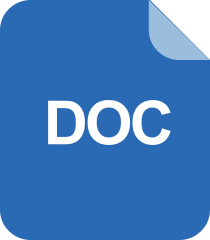
C++代码优化方案.
你的代码看起来没有明显的问题,但可以考虑以下优化:
1. 使用`unordered_set`代替`vector`进行查找:使用`unordered_set`可以将查找时间从$O(n)$优化为$O(1)$,因为`unordered_set`使用哈希表进行实现。可以将`v`定义为`unordered_set<int>`类型,并且在第一个`if`语句中使用`insert`函数来插入元素。在第二个`if`语句中使用`count`函数来判断元素是否存在。
2. 使用`cin.tie(NULL)`和`ios_base::sync_with_stdio(false)`优化输入和输出速度:这两个函数可以加速输入和输出操作,特别是在大数据量的情况下。
3. 将`using namespace std`改为使用具体的命名空间:虽然`using namespace std`可以方便我们使用`std`命名空间中的函数和对象,但是在大型项目中可能会出现命名冲突等问题,建议使用具体的命名空间。
4. 使用`auto`关键字简化代码:使用`auto`关键字可以根据变量的初始化值自动推导出变量类型,可以简化代码。
下面是优化后的代码:
```cpp
#include <bits/stdc++.h>
using std::cin;
using std::cout;
using std::endl;
using std::unordered_set;
int main() {
cin.tie(NULL);
ios_base::sync_with_stdio(false);
int n, m, k;
unordered_set<int> s;
cin >> n;
while (n--) {
cin >> m >> k;
if (m == 1) {
s.insert(k);
} else if (m == 2) {
cout << (s.count(k) ? "True" : "False") << endl;
}
}
return 0;
}
```
阅读全文
相关推荐
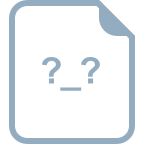
















