vue3动态增减标签页
时间: 2023-07-21 21:09:34 浏览: 56
要动态增减标签页,可以使用 Vue3 的动态组件和路由组合来实现。以下是一个简单的示例:
首先,在路由配置文件中定义动态路由:
```javascript
import { createRouter, createWebHashHistory } from 'vue-router'
const routes = [
{
path: '/',
redirect: '/home'
},
{
path: '/home',
component: () => import('@/views/Home.vue')
},
{
path: '/dynamic/:id',
name: 'dynamic',
component: () => import('@/views/Dynamic.vue')
}
]
const router = createRouter({
history: createWebHashHistory(),
routes
})
export default router
```
在上面的代码中,我们定义了一个名为 `dynamic` 的动态路由,它包含一个参数 `id`。接下来,我们需要在 `Home.vue` 组件中添加一个按钮,点击该按钮可以动态增加一个标签页:
```html
<template>
<div>
<button @click="addTab">Add Tab</button>
<div class="tabs">
<div v-for="(tab, index) in tabs" :key="tab.id" class="tab" :class="{ active: activeTab === index }">
<router-link :to="{ name: 'dynamic', params: { id: tab.id } }">{{ tab.title }}</router-link>
<span class="close" @click="closeTab(index)">x</span>
</div>
</div>
<router-view></router-view>
</div>
</template>
<script>
export default {
data() {
return {
tabs: [{ id: 1, title: 'Tab 1' }],
activeTab: 0
}
},
methods: {
addTab() {
const newTab = { id: this.tabs.length + 1, title: `Tab ${this.tabs.length + 1}` }
this.tabs.push(newTab)
this.activeTab = this.tabs.length - 1
},
closeTab(index) {
this.tabs.splice(index, 1)
if (index === this.activeTab && this.tabs.length > 0) {
this.activeTab = index === 0 ? 0 : index - 1
}
}
}
}
</script>
<style>
.tabs {
display: flex;
padding: 10px;
}
.tab {
margin-right: 10px;
cursor: pointer;
}
.tab.active {
font-weight: bold;
}
.close {
margin-left: 5px;
font-weight: bold;
color: red;
cursor: pointer;
}
</style>
```
在上面的代码中,我们使用 `v-for` 指令遍历标签页列表,并使用动态组件 `<router-view></router-view>` 来渲染动态路由组件。点击 “Add Tab” 按钮可以添加一个新的标签页,点击标签页右侧的 “x” 可以删除标签页。在 `addTab()` 方法中,我们通过 `this.$router.push()` 方法跳转到动态路由,该方法会自动匹配 `:id` 参数。在 `closeTab()` 方法中,我们根据索引删除标签页,并根据需要更新当前活动标签页的索引。
希望这个示例能帮助你实现动态增减标签页。
相关推荐
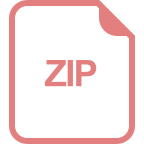
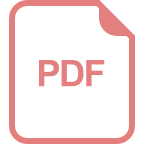














