帮我写一个实现A*算法的c++代码
时间: 2023-03-21 09:01:40 浏览: 125
好的,以下是一个简单的使用C语言实现A*算法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <math.h>
// 定义地图大小
#define ROWS 10
#define COLS 10
// 定义节点结构体
typedef struct Node {
int x; // x坐标
int y; // y坐标
int f; // f = g + h
int g; // 从起点到该点的实际代价
int h; // 从该点到终点的估计代价
struct Node *parent; // 父节点
} Node;
// 定义地图
int map[ROWS][COLS] = {
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0}
};
// 定义起点和终点
Node *start = NULL;
Node *end = NULL;
// 计算两点之间的曼哈顿距离
int manhattan_distance(int x1, int y1, int x2, int y2) {
return abs(x1 - x2) + abs(y1 - y2);
}
// 获取周围可行走的点
Node **get_neighbors(Node *current) {
int x = current->x;
int y = current->y;
Node **neighbors = malloc(8 * sizeof(Node *));
int i = 0;
for (int dx = -1; dx <= 1; dx++) {
for (int dy = -1; dy <= 1; dy++) {
if (dx == 0 && dy == 0) {
continue;
}
int nx = x + dx;
int ny = y + dy;
if (nx < 0 || nx >= ROWS || ny < 0 || ny >= COLS) {
continue;
}
if (map[nx][ny] == 1) {
continue;
}
Node *neighbor = malloc(sizeof(Node));
neighbor->x =
阅读全文
相关推荐
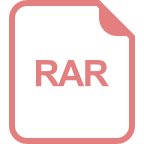
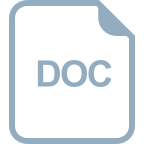
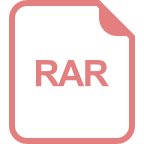
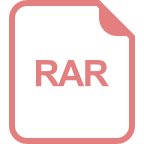
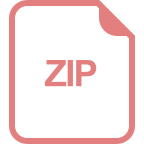
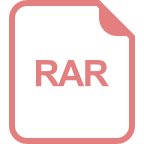
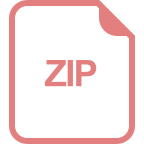
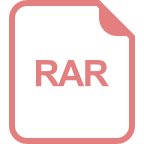
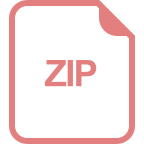
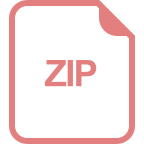
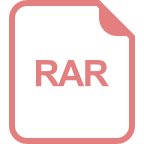
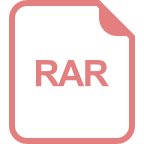