如何将mybatis的page对象转为list
时间: 2024-02-27 09:56:04 浏览: 75
Mybatis的Page对象是一个分页插件,可以将查询结果分页返回。如果你要将Mybatis的Page对象转为List,可以通过以下代码实现:
```
import com.github.pagehelper.Page;
import java.util.List;
Page<Object> page = yourPageObject;
List<Object> list = page.getResult();
```
其中,`yourPageObject`表示你需要转换的Mybatis的Page对象,`Object`表示你需要转换的对象类型。通过调用`getResult()`方法可以获取Page对象中的数据列表,然后将其转换为List类型。
需要注意的是,如果查询结果超出了分页范围,返回的Page对象中的数据列表可能为空。因此,在将Page对象转换为List之前,需要先判断Page对象中的数据是否为空。
相关问题
mybatis 遍历 对象 list
可以使用 MyBatis 的 foreach 标签来遍历对象 list。具体操作如下:
1. 在 Mapper.xml 文件中,使用 foreach 标签包裹需要遍历的 list 对象,指定集合参数和遍历时使用的对象别名。
```
<select id="selectByList" parameterType="java.util.List" resultType="com.example.User">
SELECT * FROM user WHERE id IN
<foreach collection="list" item="item" open="(" separator="," close=")">
#{item}
</foreach>
</select>
```
2. 在调用 Mapper 方法时,传入需要遍历的 list 对象。
```
List<Integer> idList = Arrays.asList(1, 2, 3);
List<User> userList = userMapper.selectByList(idList);
```
mybatis List对象
MyBatis is a popular Java-based persistence framework that provides support for mapping Java objects to SQL statements. When it comes to handling lists of objects in MyBatis, there are a few options you can consider.
1. Using the `foreach` element: MyBatis provides a `foreach` element that allows you to iterate over a collection and generate dynamic SQL statements. You can use it to handle lists of objects by specifying a collection parameter and then referencing the collection within the SQL statement. Here's an example:
```xml
<select id="getUsersByIds" parameterType="java.util.List" resultType="User">
SELECT * FROM users WHERE id IN
<foreach item="item" index="index" collection="list" open="(" separator="," close=")">
#{item}
</foreach>
</select>
```
In this example, `list` is the parameter name representing the list of object IDs.
2. Using annotations: MyBatis also supports annotations for mapping SQL statements directly to Java methods. You can use the `@Param` annotation to pass a list of objects as a parameter. Here's an example:
```java
@Select("SELECT * FROM users WHERE id IN (#{ids})")
List<User> getUsersByIds(@Param("ids") List<Integer> ids);
```
In this example, the `ids` parameter is a list of object IDs.
These are just a couple of examples of how you can handle lists of objects in MyBatis. You can choose the approach that best suits your needs and project requirements.
阅读全文
相关推荐
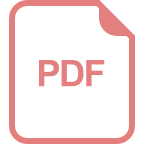
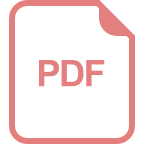
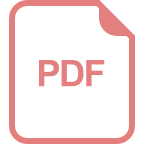













