java实现顺序栈代码
时间: 2023-11-12 14:42:25 浏览: 83
public class SeqStack<E> {
private Object[] stack; // 存储元素的数组
private int top; // 栈顶指针
private int maxSize; // 栈的容量
// 构造函数
public SeqStack(int initialSize) {
if (initialSize < 0) {
throw new IllegalArgumentException("栈初始容量不能小于0: " + initialSize);
}
this.maxSize = initialSize;
this.stack = new Object[maxSize];
this.top = -1; // 栈为空,栈顶指针为-1
}
// 判断栈是否为空
public boolean isEmpty() {
return top == -1;
}
// 判断栈是否已满
public boolean isFull() {
return top == maxSize - 1;
}
// 获取栈中元素个数
public int size() {
return top + 1;
}
// 入栈
public void push(E element) {
if (isFull()) {
throw new IndexOutOfBoundsException("栈已满,无法入栈!");
}
top++;
stack[top] = element;
}
// 出栈
public E pop() {
if (isEmpty()) {
throw new IndexOutOfBoundsException("栈为空,无法出栈!");
}
E element = (E) stack[top];
top--;
return element;
}
// 获取栈顶元素
public E peek() {
if (isEmpty()) {
return null;
}
return (E) stack[top];
}
// 清空栈
public void clear() {
top = -1;
}
}
阅读全文
相关推荐
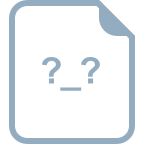
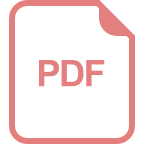
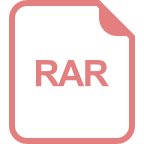
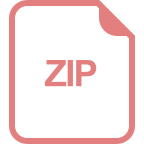
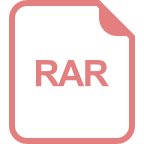
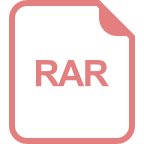

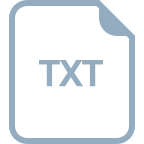
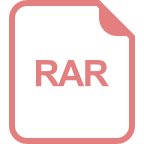
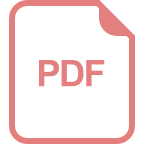
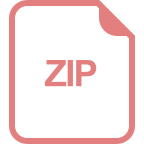
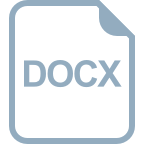
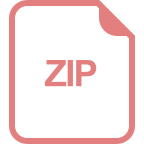
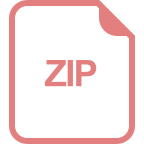
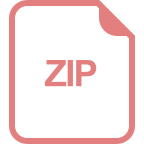
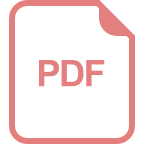
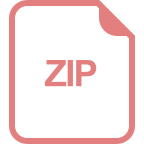