java1.设置4个线程对象,2个执行加的方法,两个执行减操作 2.设置生成计算机和搬运计算机类,生产一台搬走一台,如果没有等待生成,如果生成等待搬运,计算2分钟生成了多少台计算机 3.实现一个抢答程序,设置2个抢答者,同时发送抢答执行,答题成功提示成功,未抢答成功者提示失败
时间: 2023-10-07 21:06:04 浏览: 99
1. Java代码:
```
class AddThread implements Runnable {
private Counter counter;
public AddThread(Counter counter) {
this.counter = counter;
}
public void run() {
for (int i = 0; i < 10; i++) {
counter.add();
}
}
}
class SubThread implements Runnable {
private Counter counter;
public SubThread(Counter counter) {
this.counter = counter;
}
public void run() {
for (int i = 0; i < 10; i++) {
counter.sub();
}
}
}
class Counter {
private int count = 0;
public synchronized void add() {
count++;
System.out.println(Thread.currentThread().getName() + "加1,当前值为:" + count);
}
public synchronized void sub() {
count--;
System.out.println(Thread.currentThread().getName() + "减1,当前值为:" + count);
}
}
public class Main {
public static void main(String[] args) {
Counter counter = new Counter();
AddThread addThread1 = new AddThread(counter);
AddThread addThread2 = new AddThread(counter);
SubThread subThread1 = new SubThread(counter);
SubThread subThread2 = new SubThread(counter);
Thread thread1 = new Thread(addThread1, "线程1");
Thread thread2 = new Thread(addThread2, "线程2");
Thread thread3 = new Thread(subThread1, "线程3");
Thread thread4 = new Thread(subThread2, "线程4");
thread1.start();
thread2.start();
thread3.start();
thread4.start();
}
}
```
2. Java代码:
```
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
class Computer {
private String name;
public Computer(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
class GenerateComputerThread implements Runnable {
private BlockingQueue<Computer> queue;
public GenerateComputerThread(BlockingQueue<Computer> queue) {
this.queue = queue;
}
public void run() {
for (int i = 0; i < 10; i++) {
Computer computer = new Computer("计算机" + (i + 1));
try {
queue.put(computer);
System.out.println(Thread.currentThread().getName() + "生成了一台" + computer.getName());
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
class CarryComputerThread implements Runnable {
private BlockingQueue<Computer> queue;
public CarryComputerThread(BlockingQueue<Computer> queue) {
this.queue = queue;
}
public void run() {
while (true) {
try {
Thread.sleep(2000);
Computer computer = queue.take();
System.out.println(Thread.currentThread().getName() + "搬走了一台" + computer.getName());
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class Main {
public static void main(String[] args) {
BlockingQueue<Computer> queue = new ArrayBlockingQueue<>(10);
GenerateComputerThread generateThread = new GenerateComputerThread(queue);
CarryComputerThread carryThread = new CarryComputerThread(queue);
Thread thread1 = new Thread(generateThread, "生成计算机线程");
Thread thread2 = new Thread(carryThread, "搬运计算机线程");
thread1.start();
thread2.start();
try {
Thread.sleep(120000);
thread1.interrupt();
thread2.interrupt();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
3. Java代码:
```
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
class Answer implements Runnable {
private static int count = 0;
private static Lock lock = new ReentrantLock();
public void run() {
lock.lock();
try {
count++;
if (count == 1) {
System.out.println(Thread.currentThread().getName() + "抢答成功");
} else {
System.out.println(Thread.currentThread().getName() + "抢答失败");
}
} finally {
lock.unlock();
}
}
}
public class Main {
public static void main(String[] args) {
Answer answer = new Answer();
Thread thread1 = new Thread(answer, "抢答者1");
Thread thread2 = new Thread(answer, "抢答者2");
thread1.start();
thread2.start();
}
}
```
阅读全文
相关推荐
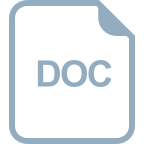
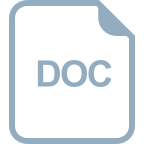
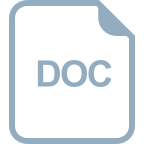
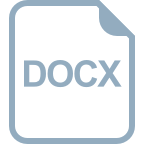
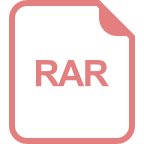
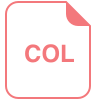

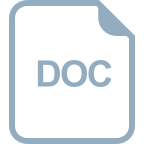
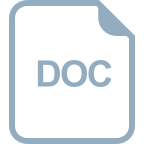
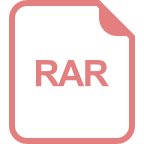
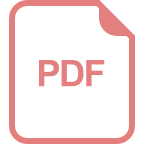
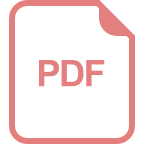
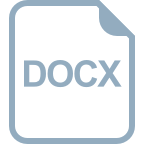
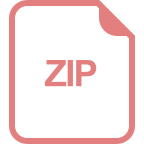
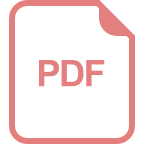
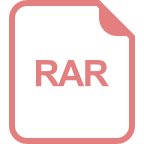
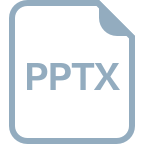
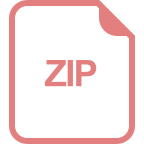