C++利用链表相关知识从头开始将若干个数字按顺序移动至字符串末端。
时间: 2024-02-24 17:00:12 浏览: 110
好的,以下是C++代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义链表节点结构体
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(nullptr) {}
};
// 将链表转换为字符串
string listToString(ListNode *head) {
string str = "";
while (head != nullptr) {
str += to_string(head->val);
head = head->next;
}
return str;
}
// 将若干个数字按顺序移动至字符串末端
string moveDigitsToEnd(string str) {
// 创建链表
ListNode *head = nullptr, *tail = nullptr;
for (char c : str) {
int digit = c - '0';
ListNode *newNode = new ListNode(digit);
if (tail == nullptr) {
head = tail = newNode;
} else {
tail->next = newNode;
tail = tail->next;
}
}
// 遍历链表,将数字按顺序移动至链表末尾
ListNode *cur = head, *prev = nullptr;
while (cur != nullptr && cur->next != nullptr) {
if (cur->val % 2 == 0) { // 当前节点为偶数,将其移动至链表末尾
ListNode *temp = cur->next;
if (prev == nullptr) { // 当前节点为头节点
head = temp;
} else {
prev->next = temp;
}
tail->next = cur;
tail = tail->next;
cur->next = nullptr;
cur = temp;
} else { // 当前节点为奇数,继续遍历
prev = cur;
cur = cur->next;
}
}
return listToString(head);
}
int main() {
string str;
cout << "Please input a string: ";
cin >> str;
str = moveDigitsToEnd(str);
cout << "After moving digits to end: " << str << endl;
return 0;
}
```
以上代码中,我们先将输入的字符串转换为数字链表。然后遍历链表,将链表中的偶数节点移动到链表末尾,保持奇数节点的相对顺序不变。最后将链表转换回字符串并输出。
阅读全文
相关推荐








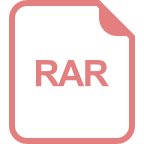
