用C语言
时间: 2024-02-16 17:04:15 浏览: 57
好的,以下是用C语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORD_LENGTH 50
typedef struct {
char word[MAX_WORD_LENGTH];
int count;
} WordCount;
int compare_word_count(const void* a, const void* b) {
int count_a = ((WordCount*)a)->count;
int count_b = ((WordCount*)b)->count;
return count_b - count_a;
}
int main() {
FILE* file = fopen("file9_1.txt", "r");
if (file == NULL) {
printf("Failed to open the file.\n");
return 1;
}
// 统计单词出现次数
int num_words = 0;
WordCount* word_counts = malloc(num_words * sizeof(WordCount));
char word[MAX_WORD_LENGTH];
while (fscanf(file, "%s", word) == 1) {
int found = 0;
for (int i = 0; i < num_words; i++) {
if (strcmp(word_counts[i].word, word) == 0) {
word_counts[i].count++;
found = 1;
break;
}
}
if (!found) {
num_words++;
word_counts = realloc(word_counts, num_words * sizeof(WordCount));
strcpy(word_counts[num_words - 1].word, word);
word_counts[num_words - 1].count = 1;
}
}
// 按出现次数排序并输出结果
qsort(word_counts, num_words, sizeof(WordCount), compare_word_count);
for (int i = 0; i < num_words; i++) {
printf("%s %d\n", word_counts[i].word, word_counts[i].count);
}
free(word_counts);
fclose(file);
return 0;
}
```
这个程序首先使用`fopen`函数打开文件,并使用`fscanf`函数逐个读取单词。对于每个单词,我们使用一个循环来查找它是否已经在`word_counts`数组中出现过,如果出现过,就将其计数器加1,否则就将其添加到数组中。最后,我们使用`qsort`函数按出现次数降序排序,并输出结果。
希望这个C语言程序可以帮助你解决问题!
阅读全文
相关推荐
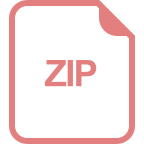
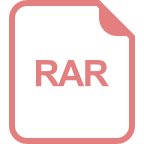
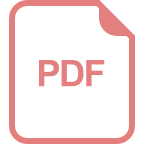