用C语言写一个状态机识别按键的短按、长按、连续短按等操作
时间: 2023-08-11 11:05:27 浏览: 61
好的,以下是一个用C语言写的状态机,用于识别按键的短按、长按、连续短按等操作:
```c
// 定义状态常量
typedef enum {
IDLE_STATE = 0,
SHORT_PRESS_STATE,
SHORT_PRESS_DONE_STATE,
LONG_PRESS_STATE,
LONG_PRESS_DONE_STATE,
CONTINUOUS_SHORT_PRESS_STATE
} buttonState_t;
// 定义全局变量
buttonState_t currentState = IDLE_STATE;
buttonState_t prevState = IDLE_STATE;
bool buttonPressed = false;
bool buttonReleased = false;
int buttonHoldTime = 0;
const int shortPressTime = 50; // 短按时间阈值
const int longPressTime = 1000; // 长按时间阈值
const int debounceTime = 10; // 消除抖动的时间
// 检测按键状态
void checkButtonState() {
if (buttonIsPressed()) {
buttonPressed = true;
buttonReleased = false;
} else if (buttonIsReleased()) {
buttonReleased = true;
buttonPressed = false;
}
}
// 消除抖动
void debounceButton() {
if (debounceTime > 0) {
delay(debounceTime);
debounceTime = 0;
}
}
// 更新按键持续时间
void updateButtonHoldTime() {
if (buttonPressed) {
buttonHoldTime++;
} else {
buttonHoldTime = 0;
}
}
// 状态转移
void transitionButtonState() {
if (currentState == IDLE_STATE) {
if (buttonPressed) {
currentState = SHORT_PRESS_STATE;
}
} else if (currentState == SHORT_PRESS_STATE) {
if (buttonReleased && buttonHoldTime < shortPressTime) {
currentState = SHORT_PRESS_DONE_STATE;
} else if (buttonHoldTime >= longPressTime) {
currentState = LONG_PRESS_STATE;
}
} else if (currentState == SHORT_PRESS_DONE_STATE) {
if (prevState == SHORT_PRESS_STATE && buttonPressed) {
currentState = DOUBLE_PRESS_STATE;
} else if (prevState == LONG_PRESS_STATE && buttonPressed) {
currentState = LONG_PRESS_DONE_STATE;
}
} else if (currentState == LONG_PRESS_STATE) {
if (buttonReleased) {
currentState = LONG_PRESS_DONE_STATE;
}
} else if (currentState == LONG_PRESS_DONE_STATE) {
if (prevState == SHORT_PRESS_STATE && buttonPressed) {
currentState = SHORT_PRESS_STATE;
} else if (buttonHoldTime >= shortPressTime) {
currentState = CONTINUOUS_SHORT_PRESS_STATE;
}
} else if (currentState == CONTINUOUS_SHORT_PRESS_STATE) {
if (buttonReleased) {
currentState = IDLE_STATE;
}
}
}
// 状态处理
void handleButtonState() {
if (currentState != prevState) {
prevState = currentState;
if (currentState == SHORT_PRESS_DONE_STATE) {
onShortPressDone();
} else if (currentState == LONG_PRESS_STATE) {
onLongPress();
} else if (currentState == LONG_PRESS_DONE_STATE) {
onLongPressDone();
} else if (currentState == DOUBLE_PRESS_STATE) {
onDoublePress();
} else if (currentState == CONTINUOUS_SHORT_PRESS_STATE) {
onContinuousShortPress();
} else if (currentState == IDLE_STATE) {
onIdle();
}
}
}
// 主函数
int main() {
while(1) {
// 检测按键状态
checkButtonState();
// 消除抖动
debounceButton();
// 更新按键持续时间
updateButtonHoldTime();
// 状态转移
transitionButtonState();
// 状态处理
handleButtonState();
}
return 0;
}
```
以上代码是一个基本的按键状态机,包含了处理短按、长按、连续短按等操作的状态。你可以根据具体的需求进行修改和优化。请确保 `buttonIsPressed()` 和 `buttonIsReleased()` 函数能够正确检测按键状态,`delay()` 函数能够正确实现延时。
相关推荐
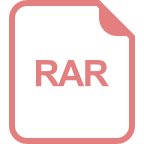
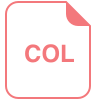
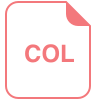
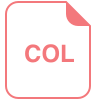
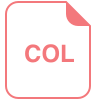
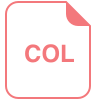









