Write c++ program. Design a Rectangle class. requirement: (1) Contains two member variables m_length and m_width, the default value of which is 1. (2) Include the member function Perimeter() to calculate the perimeter of the rectangle, and Area() to calculate the area of the rectangle. (3) The member functions setwidth() and getwidth() are used to set and get the value of m_width, and setlength() and getlength() are used to set and get the value of m_length. The Set...() function should verify that m_length and m_width are both floating numbers between 0.0 and 20.0.Wr
时间: 2024-01-05 20:03:19 浏览: 26
ite a C++ program to implement the Rectangle class.
Here's the code:
```
#include <iostream>
using namespace std;
class Rectangle {
private:
float m_length;
float m_width;
public:
Rectangle() : m_length(1), m_width(1) {} // default constructor
Rectangle(float length, float width) { setlength(length); setwidth(width); } // constructor with parameters
// member functions
float Perimeter() const { return 2 * (m_length + m_width); }
float Area() const { return m_length * m_width; }
// set and get functions
void setlength(float length) { if (length >= 0.0 && length <= 20.0) m_length = length; }
void setwidth(float width) { if (width >= 0.0 && width <= 20.0) m_width = width; }
float getlength() const { return m_length; }
float getwidth() const { return m_width; }
};
int main() {
Rectangle rect1;
Rectangle rect2(5.0, 10.0);
cout << "Rectangle 1: length = " << rect1.getlength() << ", width = " << rect1.getwidth() << endl;
cout << "Rectangle 1: perimeter = " << rect1.Perimeter() << ", area = " << rect1.Area() << endl;
cout << "Rectangle 2: length = " << rect2.getlength() << ", width = " << rect2.getwidth() << endl;
cout << "Rectangle 2: perimeter = " << rect2.Perimeter() << ", area = " << rect2.Area() << endl;
return 0;
}
```
In this program, we define a Rectangle class with two member variables `m_length` and `m_width`, and four member functions `Perimeter()`, `Area()`, `setlength()`, and `setwidth()`. The default value of `m_length` and `m_width` is 1, and the `set...()` functions verify that the values are between 0.0 and 20.0.
In the `main()` function, we create two Rectangle objects `rect1` and `rect2`, and display their dimensions, perimeter, and area.
相关推荐
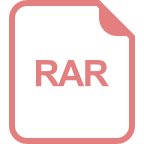
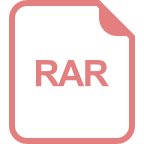














