用Python编一个程序,点击一个按钮,选择一个文件夹,在窗口中显示文件夹的内容,点击文件夹中的文件,文件的内容显示在右边的窗口中.
时间: 2024-03-01 16:51:34 浏览: 172
好,我可以帮你编写这样一个程序。我们可以使用Python自带的Tkinter库来创建窗口和按钮,并使用os库来读取文件夹的内容。以下是一个简单的程序示例:
```python
import os
import tkinter as tk
from tkinter import filedialog
root = tk.Tk()
root.title("文件管理器")
# 创建左边的文件列表框架
left_frame = tk.Frame(root)
left_frame.pack(side="left", fill="both", expand=True)
# 创建文件列表滚动条
scrollbar = tk.Scrollbar(left_frame)
scrollbar.pack(side="right", fill="y")
# 创建文件列表
file_list = tk.Listbox(left_frame, yscrollcommand=scrollbar.set)
file_list.pack(side="left", fill="both", expand=True)
scrollbar.config(command=file_list.yview)
# 创建右边的文件内容框架
right_frame = tk.Frame(root)
right_frame.pack(side="left", fill="both", expand=True)
# 创建文件内容滚动条
scrollbar2 = tk.Scrollbar(right_frame)
scrollbar2.pack(side="right", fill="y")
# 创建文件内容文本框
file_text = tk.Text(right_frame, yscrollcommand=scrollbar2.set)
file_text.pack(side="left", fill="both", expand=True)
scrollbar2.config(command=file_text.yview)
# 定义打开文件夹的函数
def open_folder():
folder_path = filedialog.askdirectory()
if folder_path:
# 清空文件列表和文件内容
file_list.delete(0, tk.END)
file_text.delete("1.0", tk.END)
# 显示文件夹中的文件
for file_name in os.listdir(folder_path):
file_list.insert(tk.END, file_name)
# 定义文件列表的点击事件
file_list.bind("<<ListboxSelect>>", show_file_content)
# 定义显示文件内容的函数
def show_file_content(event):
# 清空文件内容
file_text.delete("1.0", tk.END)
# 获取点击的文件名
file_name = file_list.get(file_list.curselection())
# 读取文件内容并显示在文本框中
with open(file_name, "r") as file:
file_content = file.read()
file_text.insert(tk.END, file_content)
# 创建打开文件夹的按钮
open_button = tk.Button(root, text="打开文件夹", command=open_folder)
open_button.pack()
root.mainloop()
```
运行这个程序,你会看到一个窗口和一个“打开文件夹”的按钮。点击按钮后,选择一个文件夹,窗口左边会显示文件夹中的文件列表,你可以点击列表中的文件名,在窗口右边的文本框中显示文件的内容。
阅读全文
相关推荐
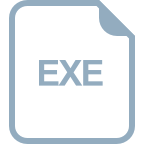















