api 句柄窗口关闭前取消窗口的所有者关系
时间: 2023-07-19 14:41:54 浏览: 47
在 Win32 API 中,可以使用 `SetWindowLong` 函数来修改窗口的所有者,从而取消窗口所有者关系。可以在 `WM_CLOSE` 消息的处理函数中取消窗口的所有者关系,从而实现在窗口关闭前取消所有者关系的功能。
具体操作步骤如下:
1. 定义窗口过程函数:
```
[DllImport("user32.dll")]
private static extern IntPtr DefWindowProc(IntPtr hWnd, uint uMsg, IntPtr wParam, IntPtr lParam);
private const uint WM_CLOSE = 0x0010;
private static IntPtr WindowProc(IntPtr hWnd, uint uMsg, IntPtr wParam, IntPtr lParam)
{
switch (uMsg)
{
case WM_CLOSE:
// 取消窗口所有者关系
SetWindowLong(hWnd, GWL_HWNDPARENT, IntPtr.Zero);
break;
default:
break;
}
return DefWindowProc(hWnd, uMsg, wParam, lParam);
}
```
其中 `WM_CLOSE` 表示窗口关闭消息,`SetWindowLong` 函数用于取消窗口所有者关系。
2. 创建窗口并设置窗口过程函数:
```
[DllImport("user32.dll")]
private static extern IntPtr CreateWindowEx(
uint dwExStyle,
string lpClassName,
string lpWindowName,
uint dwStyle,
int x,
int y,
int nWidth,
int nHeight,
IntPtr hWndParent,
IntPtr hMenu,
IntPtr hInstance,
IntPtr lpParam);
private static readonly string className = "MyClassName";
IntPtr hWnd = CreateWindowEx(
0,
className,
"MyWindow",
WS_OVERLAPPEDWINDOW,
0,
0,
640,
480,
IntPtr.Zero,
IntPtr.Zero,
IntPtr.Zero,
IntPtr.Zero);
// 设置窗口过程函数
SetWindowLong(hWnd, GWL_WNDPROC, Marshal.GetFunctionPointerForDelegate(new WindowProcDelegate(WindowProc)));
```
其中 `CreateWindowEx` 函数用于创建窗口,`SetWindowLong` 函数用于设置窗口过程函数。
3. 显示窗口并进入消息循环:
```
[DllImport("user32.dll")]
private static extern bool ShowWindow(IntPtr hWnd, int nCmdShow);
private const int SW_SHOW = 5;
ShowWindow(hWnd, SW_SHOW);
MSG msg;
while (GetMessage(out msg, IntPtr.Zero, 0, 0))
{
TranslateMessage(ref msg);
DispatchMessage(ref msg);
}
```
其中 `ShowWindow` 函数用于显示窗口,`GetMessage`、`TranslateMessage` 和 `DispatchMessage` 函数用于进入消息循环。
这样,在窗口关闭前就可以取消窗口的所有者关系。需要注意的是,取消所有者关系可能会导致子窗口无法正常显示,因此建议在确定不需要窗口所有者关系时再进行取消操作。
相关推荐
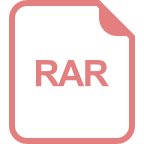
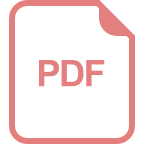
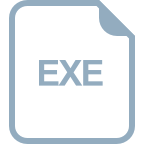














