Tensorflow搭建LSTM神经网络
时间: 2023-11-09 12:08:43 浏览: 107
1. 导入Tensorflow库和其他必要的库
```python
import tensorflow as tf
import numpy as np
```
2. 定义超参数
```python
num_epochs = 50
batch_size = 32
learning_rate = 0.001
num_classes = 2
hidden_size = 128
timesteps = 28
num_layers = 2
```
3. 加载数据集
```python
from tensorflow.examples.tutorials.mnist import input_data
mnist = input_data.read_data_sets("/tmp/data/", one_hot=True)
```
4. 定义输入和输出
```python
X = tf.placeholder("float", [None, timesteps, 28])
Y = tf.placeholder("float", [None, num_classes])
```
5. 定义LSTM模型
```python
def LSTM(x, weights, biases):
x = tf.transpose(x, [1, 0, 2])
x = tf.reshape(x, [-1, 28])
x = tf.split(x, timesteps, 0)
lstm_cell = tf.contrib.rnn.BasicLSTMCell(hidden_size, forget_bias=1.0, state_is_tuple=True)
stacked_lstm = tf.contrib.rnn.MultiRNNCell([lstm_cell] * num_layers, state_is_tuple=True)
outputs, states = tf.contrib.rnn.static_rnn(stacked_lstm, x, dtype=tf.float32)
return tf.matmul(outputs[-1], weights['out']) + biases['out']
```
6. 初始化权重和偏置
```python
weights = {
'out': tf.Variable(tf.random_normal([hidden_size, num_classes]))
}
biases = {
'out': tf.Variable(tf.random_normal([num_classes]))
}
```
7. 构建模型
```python
logits = LSTM(X, weights, biases)
prediction = tf.nn.softmax(logits)
```
8. 定义损失函数和优化器
```python
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=logits, labels=Y))
optimizer = tf.train.GradientDescentOptimizer(learning_rate=learning_rate).minimize(loss)
```
9. 定义准确率
```python
correct_pred = tf.equal(tf.argmax(prediction, 1), tf.argmax(Y, 1))
accuracy = tf.reduce_mean(tf.cast(correct_pred, tf.float32))
```
10. 训练模型
```python
init = tf.global_variables_initializer()
with tf.Session() as sess:
sess.run(init)
for epoch in range(num_epochs):
avg_loss = 0.
total_batch = int(mnist.train.num_examples/batch_size)
for i in range(total_batch):
batch_x, batch_y = mnist.train.next_batch(batch_size=batch_size)
batch_x = batch_x.reshape((batch_size, timesteps, 28))
_, loss_ = sess.run([optimizer, loss], feed_dict={X: batch_x, Y: batch_y})
avg_loss += loss_ / total_batch
if epoch % 5 == 0:
acc = sess.run(accuracy, feed_dict={X: batch_x, Y: batch_y})
print("Epoch:", '%04d' % (epoch+1), "loss={:.6f}".format(avg_loss), "accuracy={:.6f}".format(acc))
print("Optimization Finished!")
test_data = mnist.test.images.reshape((-1, timesteps, 28))
test_label = mnist.test.labels
print("Testing Accuracy:", sess.run(accuracy, feed_dict={X: test_data, Y: test_label}))
```
以上是使用Tensorflow搭建LSTM神经网络的步骤,可以根据实际需求进行调整和修改。
阅读全文
相关推荐
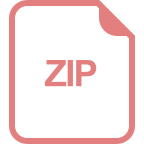

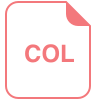
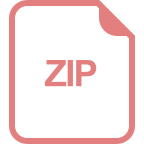
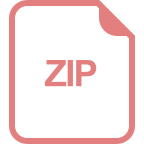
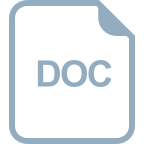

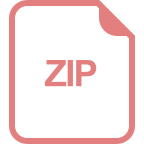
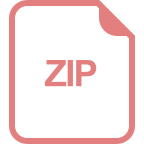
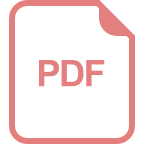
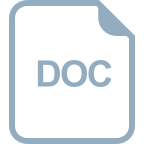
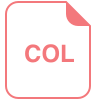
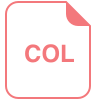
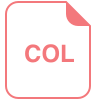
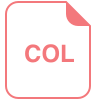

