操作系统银行家算法c语言代码
时间: 2023-10-20 12:06:36 浏览: 82
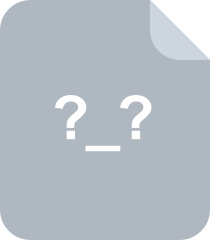
操作系统之银行家算法C代码
下面是一个简单的银行家算法的C语言代码实现:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_PROCESS_NUM 10
#define MAX_RESOURCE_NUM 10
int available[MAX_RESOURCE_NUM] = {0};
int max[MAX_PROCESS_NUM][MAX_RESOURCE_NUM] = {0};
int allocation[MAX_PROCESS_NUM][MAX_RESOURCE_NUM] = {0};
int need[MAX_PROCESS_NUM][MAX_RESOURCE_NUM] = {0};
bool finish[MAX_PROCESS_NUM] = {false};
int safe_sequence[MAX_PROCESS_NUM] = {0};
int process_num, resource_num;
bool safety_check() {
int work[MAX_RESOURCE_NUM];
bool finish_copy[MAX_PROCESS_NUM];
for (int i = 0; i < resource_num; i++) {
work[i] = available[i];
}
for (int i = 0; i < process_num; i++) {
finish_copy[i] = finish[i];
}
int count = 0;
while (count < process_num) {
bool found = false;
for (int i = 0; i < process_num; i++) {
if (!finish_copy[i]) {
bool enough = true;
for (int j = 0; j < resource_num; j++) {
if (need[i][j] > work[j]) {
enough = false;
break;
}
}
if (enough) {
found = true;
for (int j = 0; j < resource_num; j++) {
work[j] += allocation[i][j];
}
finish_copy[i] = true;
safe_sequence[count] = i;
count++;
}
}
}
if (!found) {
return false;
}
}
return true;
}
int main() {
printf("Enter the number of processes: ");
scanf("%d", &process_num);
printf("Enter the number of resources: ");
scanf("%d", &resource_num);
printf("Enter the available amount of each resource:\n");
for (int i = 0; i < resource_num; i++) {
scanf("%d", &available[i]);
}
printf("Enter the maximum demand of each process:\n");
for (int i = 0; i < process_num; i++) {
for (int j = 0; j < resource_num; j++) {
scanf("%d", &max[i][j]);
}
}
printf("Enter the amount of each resource currently allocated to each process:\n");
for (int i = 0; i < process_num; i++) {
for (int j = 0; j < resource_num; j++) {
scanf("%d", &allocation[i][j]);
need[i][j] = max[i][j] - allocation[i][j];
}
}
if (safety_check()) {
printf("Safe sequence:");
for (int i = 0; i < process_num; i++) {
printf(" P%d", safe_sequence[i]);
}
printf("\n");
} else {
printf("Unsafe state detected.\n");
}
return 0;
}
```
注意:本代码实现仅供参考,实际应用中需要根据具体情况进行修改和完善。
阅读全文
相关推荐


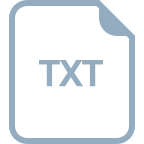
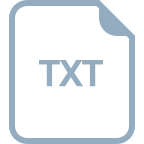
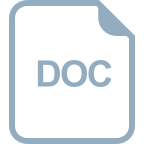
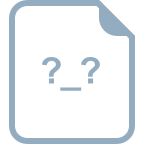
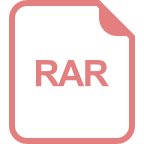
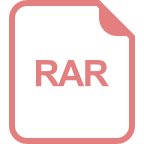
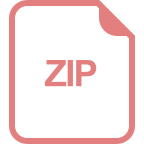
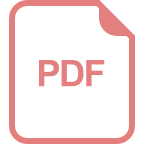



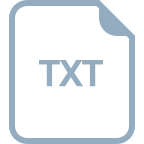