type FriendList struct { gorm.Model UserID int FriendID int State string Group []FriendGroup `gorm:"foreignKey:GroupID"` // 分组 GroupID int } type FriendGroup struct { }该怎么写呢我要实现加好友后会把好友放进默认的组,用户可以请求接口把用户进行分组自定义组名使用的是gin框架
时间: 2024-03-10 07:47:32 浏览: 12
可以按照以下方式定义`FriendList`和`FriendGroup`模型:
```go
type FriendList struct {
gorm.Model
UserID int
FriendID int
State string
Group FriendGroup `gorm:"foreignKey:GroupID"` // 分组
GroupID int
}
type FriendGroup struct {
gorm.Model
UserID int
Name string
Friends []FriendList
}
```
其中,`FriendList`和`FriendGroup`分别对应好友关系表和好友分组表。`gorm.Model`包含了`ID`、`CreatedAt`、`UpdatedAt`、`DeletedAt`等常用字段,用于简化模型定义。
`UserID`和`FriendID`分别表示用户ID和好友ID,`State`表示好友关系状态(如已添加、已删除等),`Group`表示好友所属的分组,`GroupID`表示分组ID。
`FriendGroup`模型中,`Name`表示分组名称,`Friends`表示该分组中的好友列表。
在进行好友添加操作时,可以先将好友添加到默认分组中:
```go
func addFriend(c *gin.Context) {
userID := c.GetInt("userID")
friendID := c.GetInt("friendID")
// 添加好友到默认分组
friendList := &FriendList{
UserID: userID,
FriendID: friendID,
State: "已添加",
GroupID: 0, // 默认分组ID为0
}
if err := db.Create(friendList).Error; err != nil {
c.JSON(http.StatusInternalServerError, gin.H{"error": "添加好友失败"})
return
}
c.JSON(http.StatusOK, gin.H{"message": "添加好友成功"})
}
```
在进行好友分组操作时,可以先创建新的分组,并将好友从原来的分组中移动到新的分组中:
```go
func createFriendGroup(c *gin.Context) {
userID := c.GetInt("userID")
name := c.PostForm("name")
// 创建新的分组
group := &FriendGroup{
UserID: userID,
Name: name,
}
if err := db.Create(group).Error; err != nil {
c.JSON(http.StatusInternalServerError, gin.H{"error": "创建分组失败"})
return
}
// 将好友从原来的分组中移动到新的分组中
groupID := group.ID
friendIDs := c.PostFormArray("friendIDs")
if len(friendIDs) > 0 {
if err := db.Model(&FriendList{}).
Where("user_id = ? AND friend_id IN ?", userID, friendIDs).
Updates(map[string]interface{}{"group_id": groupID}).Error; err != nil {
c.JSON(http.StatusInternalServerError, gin.H{"error": "移动好友失败"})
return
}
}
c.JSON(http.StatusOK, gin.H{"message": "创建分组成功"})
}
```
以上是一个简单的示例,具体的实现方式还需要根据具体的业务需求和框架进行调整。
相关推荐
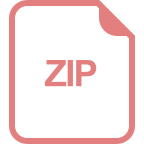














