多线程遍历怎么实现?
时间: 2024-02-12 15:04:22 浏览: 155
多线程遍历可以将文件夹分成多个小段,每个线程遍历其中一段,最后合并结果。以下是一个示例代码:
```java
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.*;
public class MultiThreadTraverseFolder {
public static void main(String[] args) {
String folderPath = "path/to/folder";
int threadCount = Runtime.getRuntime().availableProcessors();
ExecutorService executor = Executors.newFixedThreadPool(threadCount);
List<Future<List<String>>> futures = new ArrayList<>();
File folder = new File(folderPath);
if (folder.isDirectory()) {
File[] files = folder.listFiles();
if (files != null) {
int step = files.length / threadCount;
for (int i = 0; i < threadCount; i++) {
int start = i * step;
int end = (i == threadCount - 1) ? files.length : (i + 1) * step;
Callable<List<String>> task = new TraverseTask(files, start, end);
Future<List<String>> future = executor.submit(task);
futures.add(future);
}
}
}
List<String> fileList = new ArrayList<>();
for (Future<List<String>> future : futures) {
try {
fileList.addAll(future.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
}
executor.shutdown();
for (String file : fileList) {
System.out.println(file);
}
}
static class TraverseTask implements Callable<List<String>> {
private final File[] files;
private final int start;
private final int end;
public TraverseTask(File[] files, int start, int end) {
this.files = files;
this.start = start;
this.end = end;
}
@Override
public List<String> call() throws Exception {
List<String> fileList = new ArrayList<>();
for (int i = start; i < end; i++) {
File file = files[i];
if (file.isDirectory()) {
fileList.addAll(traverseFolder(file));
} else {
fileList.add(file.getAbsolutePath());
}
}
return fileList;
}
private List<String> traverseFolder(File folder) {
List<String> fileList = new ArrayList<>();
File[] files = folder.listFiles();
if (files != null) {
for (File file : files) {
if (file.isDirectory()) {
fileList.addAll(traverseFolder(file));
} else {
fileList.add(file.getAbsolutePath());
}
}
}
return fileList;
}
}
}
```
在代码中,我们首先获取 CPU 核心数,然后创建一个线程池来执行遍历任务。将文件夹分成多个小段,每个线程遍历其中一段,最后将每个线程的结果合并到一个列表中。最后输出所有文件名。在遍历过程中,如果遇到一个子文件夹,就递归调用 `traverseFolder` 方法来遍历这个子文件夹。如果遇到一个文件,就输出文件名。
需要注意的是,在多线程遍历中,要处理好线程间的同步和互斥问题,避免出现资源竞争和数据不一致的问题。
阅读全文
相关推荐
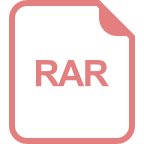
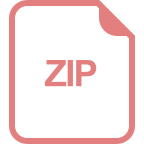
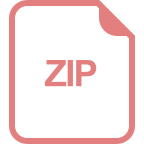
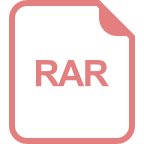
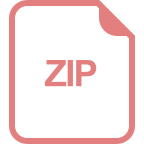
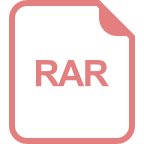
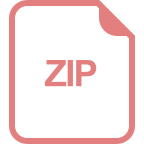
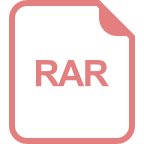
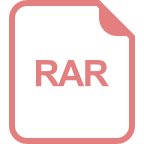
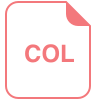






