使用java swing包写一个堆栈程序,要求实现以下功能:1.窗体上方有push和pop按钮,push按钮用于由用户输入数据。2.在窗体中间实现入栈和出栈的图形动态变化。3.堆栈的方向是由下往上
时间: 2024-02-12 12:08:46 浏览: 119
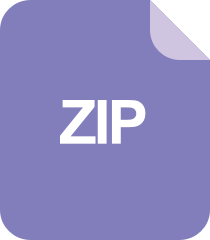
JAVA gui swing程序设计
的。4.窗体下方有一个文本框用于显示栈中的数据。5.当栈为空时,pop按钮不可用。
实现如下:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class StackProgram extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JButton pushButton, popButton;
private JTextField inputField, outputField;
private JPanel stackPanel;
private int top = -1;
private int[] stack = new int[5];
private JLabel[] stackLabels = new JLabel[5];
public StackProgram() {
setTitle("Stack Program");
setSize(300, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
pushButton = new JButton("Push");
pushButton.addActionListener(this);
popButton = new JButton("Pop");
popButton.addActionListener(this);
popButton.setEnabled(false);
inputField = new JTextField(10);
outputField = new JTextField(10);
outputField.setEditable(false);
JPanel topPanel = new JPanel();
topPanel.add(pushButton);
topPanel.add(popButton);
topPanel.add(inputField);
topPanel.add(outputField);
stackPanel = new JPanel();
stackPanel.setLayout(new GridLayout(5, 1));
for (int i = 0; i < 5; i++) {
stackLabels[i] = new JLabel("");
stackPanel.add(stackLabels[i]);
}
add(topPanel, BorderLayout.NORTH);
add(stackPanel, BorderLayout.CENTER);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == pushButton) {
if (top == 4) {
JOptionPane.showMessageDialog(null, "Stack is full!");
return;
}
top++;
stack[top] = Integer.parseInt(inputField.getText());
inputField.setText("");
popButton.setEnabled(true);
updateStackLabels();
} else if (e.getSource() == popButton) {
if (top == -1) {
JOptionPane.showMessageDialog(null, "Stack is empty!");
popButton.setEnabled(false);
return;
}
top--;
outputField.setText("" + stack[top + 1]);
updateStackLabels();
if (top == -1) {
popButton.setEnabled(false);
}
}
}
private void updateStackLabels() {
for (int i = 0; i < 5; i++) {
if (i <= top) {
stackLabels[i].setText("" + stack[top - i]);
} else {
stackLabels[i].setText("");
}
}
}
public static void main(String[] args) {
new StackProgram();
}
}
```
这是一个比较简单的堆栈程序,使用了Java Swing包来实现图形界面。在窗体上方有“Push”和“Pop”按钮,用户可以通过“Push”按钮将数据压入堆栈中,通过“Pop”按钮将数据从堆栈中弹出。在窗体中央用5个标签来显示堆栈,堆栈的方向是由下往上的。窗体下方有一个文本框用于显示栈中的数据。当栈为空时,pop按钮不可用。
阅读全文
相关推荐
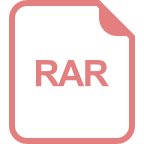
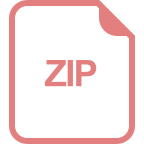
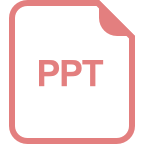
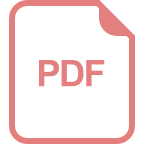
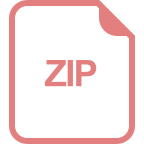
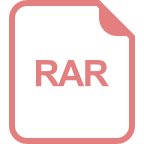
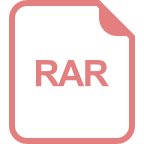
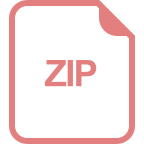
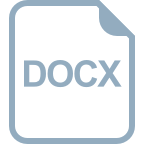
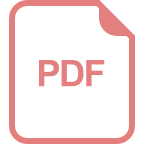
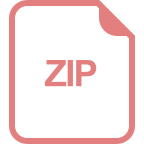
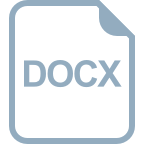
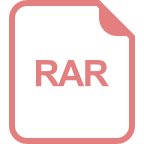
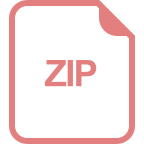
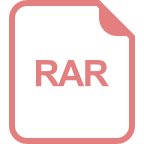
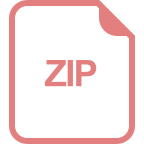
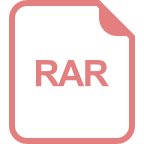