用C++代码写一个IEEE754标准的16进制数转浮点的代码
时间: 2023-06-07 20:10:02 浏览: 115
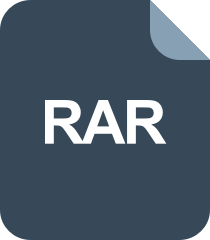
ieee754100.rar_Internet/IE编程_Visual_C++_
以下是实现IEEE754标准的16进制数转浮点的C代码示例:
```
#include <stdio.h>
#include <math.h>
union {
float f;
unsigned int i;
} num;
void hexToFloat(char hex[8]) {
num.i = (unsigned int) strtol(hex, NULL, 16);
printf("Float value: %f\n", num.f);
}
int main() {
char hex[8];
printf("Enter 16-digit hexadecimal number: ");
scanf("%s", hex);
hexToFloat(hex);
return 0;
}
```
此代码可以将16进制数转换为浮点数,并打印出转换后的值。若需要实现双精度浮点数的转换,请将float类型改为double类型。
阅读全文
相关推荐
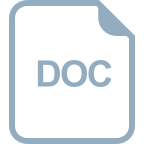
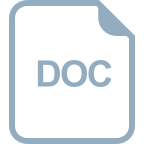

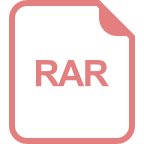
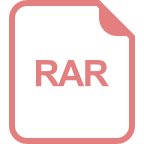
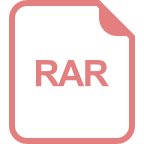

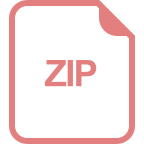
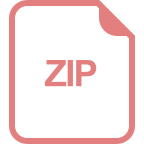
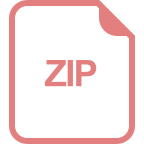
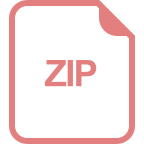
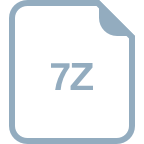

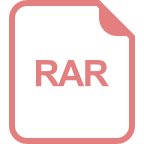
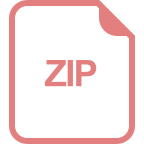
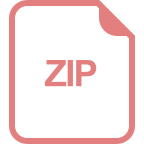
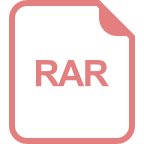
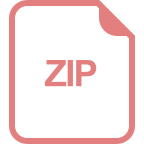