#include <iostream> using namespace std; class Location { private: int X,Y; public: void init(int initX,int initY) { X=initX; Y=initY; } int sunXY() { return X+Y; } }; int main() { Location A1; int x,y; A1.init(5,3); x=A1.X;y=A1.Y;//main 函数不能访问对象A1的私有成员 cout<<x+y<<" "<<A1.sumXY()<<endl; return 0; }以上代码有何错误,指出并修改
时间: 2024-01-09 12:05:28 浏览: 52
以上代码中的错误是在main函数中尝试访问对象A1的私有成员X和Y。私有成员只能在类的成员函数中被访问,无法在类的外部被访问。
修改后的代码如下:
```
#include <iostream>
using namespace std;
class Location {
private:
int X,Y;
public:
void init(int initX,int initY) {
X=initX;
Y=initY;
}
int sumXY() {
return X+Y;
}
// 添加 getX 和 getY 成员函数以便访问私有成员
int getX() {
return X;
}
int getY() {
return Y;
}
};
int main() {
Location A1;
int x,y;
A1.init(5,3);
x=A1.getX(); // 通过getX访问私有成员X
y=A1.getY(); // 通过getY访问私有成员Y
cout<<x+y<<" "<<A1.sumXY()<<endl;
return 0;
}
```
相关问题
补全程序,并写出程序运行结果。 #include <iostream> using namespace std; class Class { public : Class ( int...
补全程序如下:
```
#include <iostream>
using namespace std;
class Class {
public:
Class(int a, int b, int c) {
x = a;
y = b;
z = c;
}
void print() {
cout << "x: " << x << endl;
cout << "y: " << y << endl;
cout << "z: " << z << endl;
}
private:
int x;
int y;
int z;
};
int main() {
Class obj(1, 2, 3);
obj.print();
return 0;
}
```
程序运行结果为:
```
x: 1
y: 2
z: 3
```
程序定义了一个名为 Class 的类,该类包括有参构造函数和一个 print() 函数,构造函数用于对类的私有成员变量进行初始化,print() 函数用于输出类的私有成员变量。在主函数 main() 中,创建了一个名为 obj 的 Class 类对象,构造函数传入三个整数参数 1、2 和 3。最后调用 obj 对象的 print() 函数,输出 obj 对象的私有成员变量 x、y 和 z 的值。
#include<iostream>using namespace std;
这是一个C++的代码片段,它包含了iostream头文件和使用了命名空间std。iostream头文件提供了输入输出流的功能,而using namespace std;语句则是为了方便使用std命名空间中的函数和对象,避免每次都需要写std::。
以下是一个简单的示例代码,展示了如何使用iostream头文件和命名空间std来输出一句话:
```cpp
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
```
这段代码会输出"Hello, World!",endl是一个特殊的控制符,用于在输出流中插入一个换行符。
相关推荐
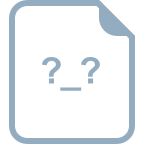












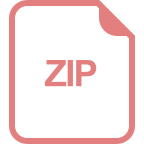
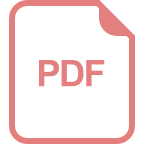