在MyBatis-Plus集成的IDEA中编写学情分析代码,通过查询数据库中的成绩信息,计算每个学生的平均分、最高分、最低分等数据,
时间: 2024-01-21 21:17:40 浏览: 35
可以按照以下步骤操作:
1. 在IDEA中创建一个MyBatis-Plus项目,并导入相关依赖。
2. 创建一个实体类,用来映射数据库中的成绩表,并使用注解来标记表名和字段名。
3. 创建一个Mapper接口,用来定义查询成绩信息的方法。可以使用MyBatis-Plus提供的方法,也可以自定义SQL语句。
4. 在Mapper接口中定义一个方法,用来查询所有学生的成绩信息,并返回一个List集合。
5. 在Service层中调用Mapper接口中定义的方法,获取所有学生的成绩信息。
6. 编写计算平均分、最高分、最低分等数据的代码,并将结果保存到一个Map中。
7. 在Controller层中将结果返回给前端页面进行展示。
下面是一个示例代码:
```java
//实体类
@Data
public class Score {
@TableId(type = IdType.AUTO)
private Long id;
private Long studentId;
private String subject;
private Integer score;
}
//Mapper接口
public interface ScoreMapper extends BaseMapper<Score> {
List<Score> selectAllScores();
}
//Service层
@Service
public class ScoreService {
@Autowired
private ScoreMapper scoreMapper;
public Map<Long, Map<String, Object>> getAnalysisData() {
List<Score> scoreList = scoreMapper.selectAllScores();
Map<Long, Map<String, Object>> analysisMap = new HashMap<>();
for (Score score : scoreList) {
Long studentId = score.getStudentId();
if (!analysisMap.containsKey(studentId)) {
analysisMap.put(studentId, new HashMap<>());
analysisMap.get(studentId).put("totalScore", 0);
analysisMap.get(studentId).put("maxScore", 0);
analysisMap.get(studentId).put("minScore", 100);
analysisMap.get(studentId).put("subjectCount", 0);
}
int totalScore = (int) analysisMap.get(studentId).get("totalScore");
int maxScore = (int) analysisMap.get(studentId).get("maxScore");
int minScore = (int) analysisMap.get(studentId).get("minScore");
int subjectCount = (int) analysisMap.get(studentId).get("subjectCount");
totalScore += score.getScore();
maxScore = Math.max(score.getScore(), maxScore);
minScore = Math.min(score.getScore(), minScore);
subjectCount++;
analysisMap.get(studentId).put("totalScore", totalScore);
analysisMap.get(studentId).put("maxScore", maxScore);
analysisMap.get(studentId).put("minScore", minScore);
analysisMap.get(studentId).put("subjectCount", subjectCount);
}
for (Map.Entry<Long, Map<String, Object>> entry : analysisMap.entrySet()) {
int totalScore = (int) entry.getValue().get("totalScore");
int subjectCount = (int) entry.getValue().get("subjectCount");
int avgScore = totalScore / subjectCount;
entry.getValue().put("avgScore", avgScore);
}
return analysisMap;
}
}
//Controller层
@RestController
@RequestMapping("/score")
public class ScoreController {
@Autowired
private ScoreService scoreService;
@GetMapping("/analysis")
public Map<Long, Map<String, Object>> getAnalysisData() {
return scoreService.getAnalysisData();
}
}
```
在上面的代码中,首先定义了一个Score实体类,用来映射数据库中的成绩表。然后定义了一个ScoreMapper接口,用来查询成绩信息。在ScoreService中编写了计算平均分、最高分、最低分等数据的代码,并将结果保存到一个Map中。最后,在ScoreController中将结果返回给前端页面进行展示。
相关推荐
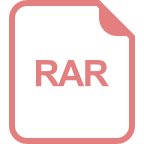
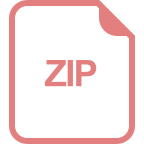














