ExplorerBar怎样使用
时间: 2024-02-21 14:35:14 浏览: 109
ExplorerBar 是一个 Windows API 控件,它可以在 Windows 窗口中创建一个具有树形结构的导航栏,类似于 Windows 资源管理器中的导航栏。要使用 ExplorerBar 控件,您需要完成以下步骤:
1. 在您的 Windows 应用程序中包含 commctrl.h 头文件。
2. 调用 InitCommonControls() 函数以初始化公共控件。
3. 创建一个父窗口,并在其中创建一个子窗口,该子窗口将承载 ExplorerBar 控件。
4. 调用 CreateWindowEx() 函数来创建 ExplorerBar 控件。
5. 使用 ExplorerBar_AddItem() 函数添加项目到控件中。
6. 为每个项目设置属性,例如图标、文本和状态。
7. 使用 ExplorerBar_Expand() 函数展开或折叠项目。
8. 处理 WM_NOTIFY 消息以响应用户与控件的交互。
下面是一个简单的示例代码,演示如何使用 ExplorerBar 控件:
```c++
#include <windows.h>
#include <commctrl.h>
#pragma comment(lib, "comctl32.lib")
LRESULT CALLBACK WndProc(HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam)
{
switch (message)
{
case WM_CREATE:
{
// 初始化公共控件
InitCommonControls();
// 创建 ExplorerBar 控件
HWND hExplorerBar = CreateWindowEx(0, WC_EXPLORER, NULL, WS_CHILD | WS_VISIBLE | WS_CLIPSIBLINGS | WS_CLIPCHILDREN, 0, 0, 200, 300, hWnd, NULL, GetModuleHandle(NULL), NULL);
// 添加项目
EXPLORERITEM ei = { 0 };
ei.mask = EIM_TEXT | EIM_CHILDREN;
ei.pszText = TEXT("Item 1");
ei.cChildren = 2;
SendMessage(hExplorerBar, EM_INSERTITEM, 0, (LPARAM)&ei);
ei.pszText = TEXT("Item 2");
ei.cChildren = 0;
SendMessage(hExplorerBar, EM_INSERTITEM, 0, (LPARAM)&ei);
ei.pszText = TEXT("Item 3");
ei.cChildren = 0;
SendMessage(hExplorerBar, EM_INSERTITEM, 0, (LPARAM)&ei);
// 展开第一个项目
ExplorerBar_Expand(hExplorerBar, 0, TRUE);
}
return 0;
case WM_NOTIFY:
{
LPNMHDR lpnm = (LPNMHDR)lParam;
switch (lpnm->code)
{
case NM_CLICK:
{
LPNMLISTVIEW lpnmlv = (LPNMLISTVIEW)lParam;
if (lpnmlv->hdr.hwndFrom == GetDlgItem(hWnd, IDC_EXPLORERBAR))
{
// 处理 ExplorerBar 点击事件
}
}
break;
}
}
return 0;
case WM_DESTROY:
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hWnd, message, wParam, lParam);
}
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
// 注册窗口类
WNDCLASSEX wcex = { 0 };
wcex.cbSize = sizeof(WNDCLASSEX);
wcex.lpfnWndProc = WndProc;
wcex.hInstance = hInstance;
wcex.hCursor = LoadCursor(NULL, IDC_ARROW);
wcex.lpszClassName = TEXT("ExplorerBarDemo");
RegisterClassEx(&wcex);
// 创建窗口
HWND hWnd = CreateWindow(TEXT("ExplorerBarDemo"), TEXT("ExplorerBar Demo"), WS_OVERLAPPEDWINDOW, CW_USEDEFAULT, CW_USEDEFAULT, 300, 400, NULL, NULL, hInstance, NULL);
// 显示窗口
ShowWindow(hWnd, nCmdShow);
UpdateWindow(hWnd);
// 消息循环
MSG msg = { 0 };
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return (int)msg.wParam;
}
```
请注意,上述示例代码仅是一个演示,并不包含所有可能用到的功能。如果您需要更详细的信息,请查看 Microsoft MSDN 文档。
阅读全文
相关推荐
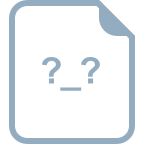
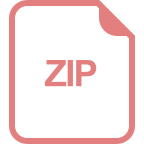
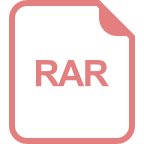
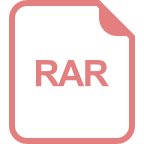
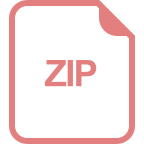
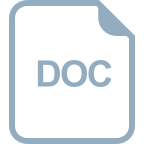
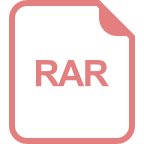
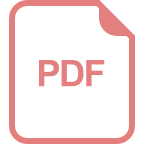
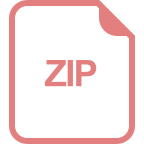
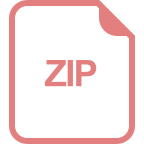
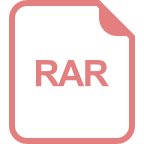
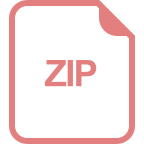
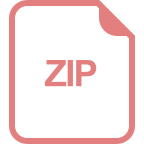
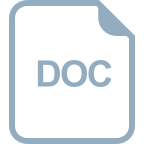

