AdaBoost的算法实现
时间: 2023-08-28 09:33:00 浏览: 92
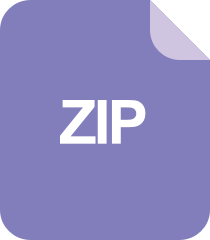
adaboost1.zip_adaboost
AdaBoost(Adaptive Boosting)是一种常见的机器学习算法,它可以将多个弱分类器组合起来形成一个强分类器。下面是AdaBoost的算法实现步骤:
1. 初始化训练数据的权值分布,使每个数据点的权值相等。
2. 对于每个弱分类器:
1) 使用当前数据权值分布对训练数据进行训练。
2) 计算分类误差率,即分类错误的样本的权值之和。
3) 计算弱分类器权重,即将分类误差率转化为分类正确率的对数。
4) 更新数据权值分布,即增加分类错误的样本的权值,减少分类正确的样本的权值。
3. 将所有弱分类器组合成一个强分类器,其中每个弱分类器的权重由它的分类正确率决定。
下面是一个简单的AdaBoost算法的Python实现:
```python
import numpy as np
class AdaBoost:
def __init__(self, n_estimators=50):
self.n_estimators = n_estimators
self.classifiers = []
self.classifier_weights = []
def fit(self, X, y):
n_samples = len(X)
sample_weights = np.ones(n_samples) / n_samples
for i in range(self.n_estimators):
classifier = DecisionTreeClassifier(max_depth=1)
classifier.fit(X, y, sample_weight=sample_weights)
y_pred = classifier.predict(X)
error = np.sum(sample_weights[y_pred != y])
if error > 0.5:
break
classifier_weight = np.log((1 - error) / error)
sample_weights *= np.exp(classifier_weight * (y_pred != y))
sample_weights /= np.sum(sample_weights)
self.classifiers.append(classifier)
self.classifier_weights.append(classifier_weight)
def predict(self, X):
y_pred = np.zeros(len(X))
for i, classifier in enumerate(self.classifiers):
y_pred += self.classifier_weights[i] * classifier.predict(X)
return np.sign(y_pred)
```
这个实现使用了sklearn库中的决策树分类器作为弱分类器,并在每次迭代中更新数据权值分布和弱分类器权重。最终将所有弱分类器的结果组合成一个强分类器。
阅读全文
相关推荐
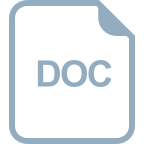
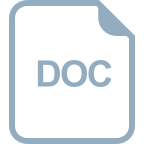
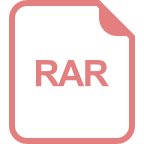
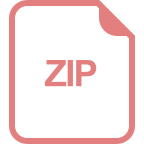

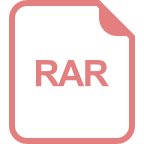
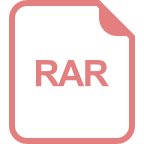
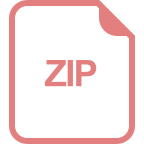
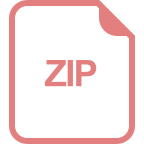
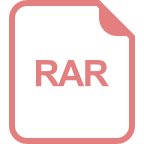
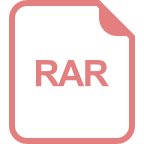
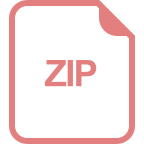
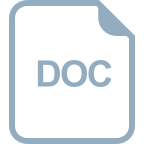
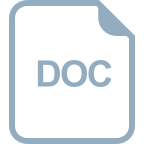
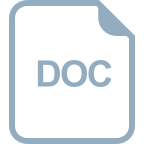
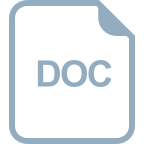
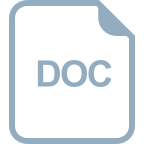
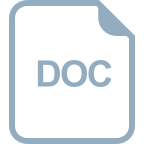