本地词向量文件加载到情感分类模型中的pytorch代码
时间: 2023-11-11 18:04:14 浏览: 98
加载本地词向量文件到情感分类模型中的PyTorch代码可以按照以下步骤进行:
1. 导入必要的库
``` python
import torch
import torch.nn as nn
import numpy as np
```
2. 定义情感分类模型
``` python
class SentimentClassifier(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim, embeddings):
super(SentimentClassifier, self).__init__()
self.embedding_dim = embeddings.shape[1]
self.embedding = nn.Embedding(input_dim, self.embedding_dim)
self.embedding.weight.data.copy_(torch.from_numpy(embeddings))
self.embedding.weight.requires_grad = False
self.hidden_dim = hidden_dim
self.lstm = nn.LSTM(self.embedding_dim, hidden_dim, batch_first=True)
self.fc = nn.Linear(hidden_dim, output_dim)
def forward(self, text, text_lengths):
embedded = self.embedding(text)
packed_embedded = nn.utils.rnn.pack_padded_sequence(embedded, text_lengths.cpu(), batch_first=True)
packed_output, (hidden, cell) = self.lstm(packed_embedded)
output, output_lengths = nn.utils.rnn.pad_packed_sequence(packed_output, batch_first=True)
hidden = self.fc(torch.mean(output, dim=1))
return hidden
```
3. 加载本地词向量文件
``` python
def load_embeddings(embedding_file):
with open(embedding_file, 'r') as f:
embeddings = {}
for line in f:
values = line.strip().split()
word = values[0]
vector = np.asarray(values[1:], dtype='float32')
embeddings[word] = vector
return embeddings
```
4. 准备数据并创建模型实例
``` python
# 准备数据
vocab_size = len(vocab)
hidden_dim = 256
output_dim = 2
embeddings_file = 'word_embeddings.txt'
embeddings = load_embeddings(embeddings_file)
# 创建模型实例
model = SentimentClassifier(vocab_size, hidden_dim, output_dim, embeddings)
```
其中,`vocab` 是一个词汇表,可以使用 PyTorch 中的 `torchtext` 库来创建。`word_embeddings.txt` 是包含每个单词的向量表示的本地文件。
5. 训练和评估模型
使用 `torch.optim` 库中的优化器来训练模型,并使用 `torch.utils.data` 库中的数据加载器来加载数据。最后,使用测试集来评估模型的性能。
``` python
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
# 定义训练循环
def train(model, iterator, optimizer, criterion):
model.train()
for batch in iterator:
text, text_lengths = batch.text
optimizer.zero_grad()
predictions = model(text, text_lengths).squeeze(1)
loss = criterion(predictions, batch.label)
loss.backward()
optimizer.step()
# 定义评估函数
def evaluate(model, iterator, criterion):
model.eval()
total_loss = 0
total_correct = 0
with torch.no_grad():
for batch in iterator:
text, text_lengths = batch.text
predictions = model(text, text_lengths).squeeze(1)
loss = criterion(predictions, batch.label)
total_loss += loss.item()
total_correct += (predictions.argmax(1) == batch.label).sum().item()
return total_loss / len(iterator), total_correct / len(iterator.dataset)
# 训练模型
N_EPOCHS = 10
for epoch in range(N_EPOCHS):
train(model, train_iterator, optimizer, criterion)
train_loss, train_acc = evaluate(model, train_iterator, criterion)
test_loss, test_acc = evaluate(model, test_iterator, criterion)
print(f'Epoch: {epoch+1:02} | Train Loss: {train_loss:.3f} | Train Acc: {train_acc*100:.2f}% | Test Loss: {test_loss:.3f} | Test Acc: {test_acc*100:.2f}%')
```
阅读全文
相关推荐
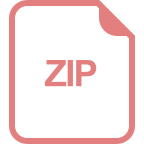
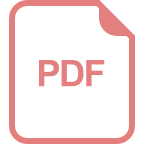



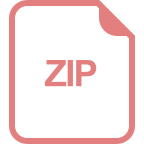
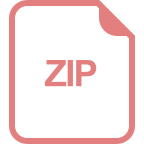
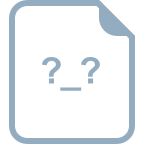
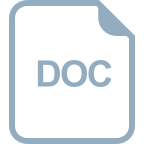
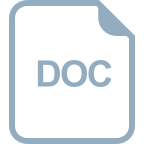
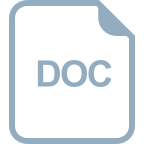
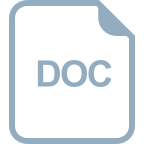
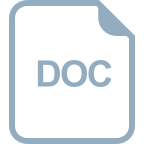
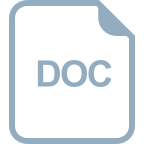
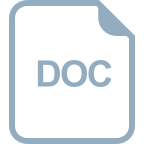
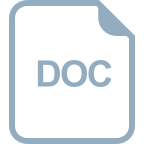

