golang对接minio
时间: 2023-10-03 18:02:11 浏览: 181
要在 Golang 中对接 MinIO,需要使用 MinIO 的 SDK。下面是一个简单的示例代码,用于上传和下载文件:
```go
package main
import (
"fmt"
"io/ioutil"
"log"
"os"
"github.com/minio/minio-go/v7"
"github.com/minio/minio-go/v7/pkg/credentials"
)
func main() {
endpoint := "play.min.io"
accessKey := "Q3AM3UQ867SPQQA43P2F"
secretKey := "zuf+tfteSlswRu7BJ86wekitnifILbZam1KYY3TG"
useSSL := true
// 初始化 MinIO 客户端对象
minioClient, err := minio.New(endpoint, &minio.Options{
Creds: credentials.NewStaticV4(accessKey, secretKey, ""),
Secure: useSSL,
})
if err != nil {
log.Fatalln(err)
}
// 检查存储桶是否存在,如果不存在则创建
bucketName := "testbucket"
exists, err := minioClient.BucketExists(context.Background(), bucketName)
if err != nil {
log.Fatalln(err)
}
if !exists {
err = minioClient.MakeBucket(context.Background(), bucketName, minio.MakeBucketOptions{})
if err != nil {
log.Fatalln(err)
}
fmt.Println("Bucket created successfully.")
}
// 上传文件
objectName := "testfile.txt"
filePath := "/path/to/testfile.txt"
contentType := "application/octet-stream"
file, err := os.Open(filePath)
if err != nil {
log.Fatalln(err)
}
defer file.Close()
_, err = minioClient.PutObject(context.Background(), bucketName, objectName, file, -1, minio.PutObjectOptions{ContentType: contentType})
if err != nil {
log.Fatalln(err)
}
fmt.Println("File uploaded successfully.")
// 下载文件
downloadFilePath := "/path/to/downloadedfile.txt"
err = minioClient.FGetObject(context.Background(), bucketName, objectName, downloadFilePath, minio.GetObjectOptions{})
if err != nil {
log.Fatalln(err)
}
fmt.Println("File downloaded successfully.")
}
```
在上面的示例中,需要替换有效的 MinIO 端点、访问密钥和存储桶名称。还需要将 `filePath` 替换为要上传的文件的路径。
阅读全文
相关推荐
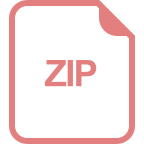
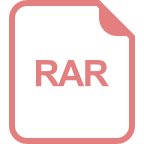















