C# 线程写入csv格式文件
时间: 2023-07-12 16:14:31 浏览: 71
以下是一个简单的示例,演示如何在 C# 中使用线程写入 CSV 格式文件:
```csharp
using System;
using System.IO;
using System.Threading;
namespace CSVWriterExample
{
class Program
{
static void Main(string[] args)
{
// 创建线程
Thread thread = new Thread(new ThreadStart(WriteToCSV));
// 启动线程
thread.Start();
Console.WriteLine("Press any key to exit.");
Console.ReadKey();
}
static void WriteToCSV()
{
// 创建一个 CSV 文件并写入标题行
string filePath = "data.csv";
using (StreamWriter writer = new StreamWriter(filePath))
{
writer.WriteLine("Name, Age, Gender");
// 模拟写入数据
for (int i = 0; i < 10; i++)
{
string name = "Person " + i;
int age = i + 20;
string gender = i % 2 == 0 ? "Male" : "Female";
// 写入数据行
writer.WriteLine("{0}, {1}, {2}", name, age, gender);
}
}
Console.WriteLine("Data written to CSV file.");
}
}
}
```
在上面的示例中,我们创建了一个名为 `WriteToCSV` 的方法,该方法模拟写入一些数据到 CSV 文件。我们使用 `StreamWriter` 类来写入文件并使用 `Thread` 类创建一个新线程来执行 `WriteToCSV` 方法。
请注意,我们在主线程中启动了新线程,并等待用户按下任意键后退出程序。这是因为我们希望新线程能够有足够的时间来完成写入操作。如果我们不等待新线程完成写入操作,那么程序可能会在写入操作完成之前退出,从而导致数据丢失。
在实际使用中,请根据您的需求修改代码,确保代码能够正确地写入 CSV 文件并处理任何可能出现的异常。
相关推荐
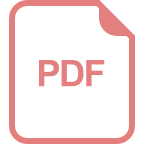














